const struct timespec
时间: 2023-10-16 12:09:57 浏览: 165
`const struct timespec` 是一个用于表示时间的结构体类型。它在 POSIX 标准中定义,用于存储绝对时间或相对时间的信息。
该结构体的定义如下:
```c
struct timespec {
time_t tv_sec; // 秒数
long tv_nsec; // 纳秒数
};
```
其中,`tv_sec` 字段表示秒数,`tv_nsec` 字段表示纳秒数。通过结合这两个字段,可以表示一个精确到纳秒级别的时间。
在多线程编程中,常常会用到 `struct timespec` 类型来指定等待的超时时间。例如,在调用 pthread_cond_timedwait 函数时,就需要传递一个 `const struct timespec` 类型的参数来指定超时时间点。
希望以上信息能够解答你的问题。如果还有任何疑问,请随时提出。
相关问题
// TODO: Move to a separate .h template <typename T> static inline const T &min(const T &x, const T &y) { return x < y ? x : y; } template <typename T> static inline const T &max(const T &x, const T &y) { return x > y ? x : y; } static inline nsecs_t framesToNanoseconds(ssize_t frames, uint32_t sampleRate, float speed) { return ((double)frames * 1000000000) / ((double)sampleRate * speed); } static int64_t convertTimespecToUs(const struct timespec &tv) { return tv.tv_sec * 1000000LL + tv.tv_nsec / 1000; } // TODO move to audio_utils. static inline struct timespec convertNsToTimespec(int64_t ns) { struct timespec tv; tv.tv_sec = static_cast<time_t>(ns / NANOS_PER_SECOND); tv.tv_nsec = static_cast<int64_t>(ns % NANOS_PER_SECOND); return tv; } // current monotonic time in microseconds. static int64_t getNowUs() { struct timespec tv; (void) clock_gettime(CLOCK_MONOTONIC, &tv); return convertTimespecToUs(tv); }
这段代码看起来像是一些常用的工具函数,包括求最小值和最大值、将帧数转换为纳秒数、将 timespec 结构体转换为微秒数、将纳秒数转换为 timespec 结构体以及获取当前的单调时间戳。这些函数可能会在音频处理等方面使用。其中,对于获取当前单调时间戳的函数,它使用了 clock_gettime 函数,并传入了 CLOCK_MONOTONIC 参数,以获得相对于系统启动时间的单调时间戳。
Windows如何替代`sys/time.h`中的`struct timespec`?
Windows操作系统中并没有直接提供类似于`sys/time.h`在Unix/Linux系统中的`struct timespec`结构体,它主要用于处理时间间隔,特别是在高精度的时间管理上。但在Windows上,你可以使用`<windows.h>`头文件中的`FILETIME`结构体来代替,它代表了自1601年1月1日开始的一个100纳秒的时间戳。
`FILETIME`通常与`ULARGE_INTEGER`一起使用,可以转换成更易操作的时间格式。例如,你可以这样做:
```cpp
#include <windows.h>
#include <iostream>
typedef ULARGE_INTEGER FILETIME_TIMESTAMP;
FILETIME_TIMESTAMP ft;
// 获取当前时间戳
void GetCurrentTime(FILETIME* pFiletime) {
GetSystemTimeAsFileTime(pFiletime);
}
// 将FILETIME转换为ticks(100纳秒单位)
DWORD64 ToTicks(const FILETIME& filetime) {
return ((filetime.dwHighDateTime << 32) | filetime.dwLowDateTime) / 10000; // 转换因子10^7
}
int main() {
FILETIME ft;
GetCurrentTime(&ft);
DWORD64 ticks = ToTicks(ft);
std::cout << "Current time in ticks: " << ticks << std::endl;
return 0;
}
```
然而,需要注意的是,Windows API对于跨线程和长时间的时间间隔计算可能不如POSIX标准中的`timespec`精确和一致。如果你需要高性能和跨平台的解决方案,可能需要使用第三方库如Boost或其他专门针对Windows开发的日期/时间库。
阅读全文
相关推荐
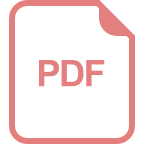
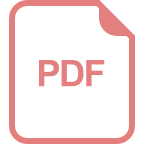
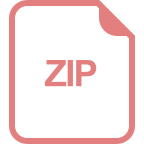


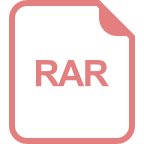
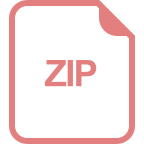
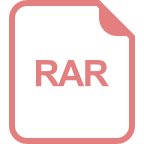
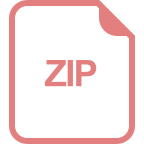