python中文文本分析例子
时间: 2023-08-04 19:07:15 浏览: 177
下面是一个简单的Python中文文本分析的例子,它可以对一段中文文本进行分词、词频统计和可视化:
```python
import jieba
from collections import Counter
import matplotlib.pyplot as plt
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 分词
words = jieba.cut(text)
# 统计词频
word_counts = Counter(words)
# 取出前10个出现频率最高的词
top_words = word_counts.most_common(10)
# 输出前10个出现频率最高的词
for word, count in top_words:
print(word, count)
# 生成词频柱状图
x = [word for word, count in top_words]
y = [count for word, count in top_words]
plt.bar(x, y)
plt.show()
```
在这个例子中,我们使用了jieba分词库来对中文文本进行分词,然后使用collections库的Counter类来统计词频。最后,我们使用matplotlib库生成了一个词频柱状图来直观展示分析结果。你可以将`text.txt`替换为你自己的文本文件来进行分析。
相关问题
python文本分析例子
以下是一个简单的Python文本分析的例子,用于计算一段文本中每个单词出现的频率:
```python
text = "This is a sample text for text analysis. Sample text is good for analysis."
# 将文本转换成小写,去除标点符号
text = text.lower()
text = text.translate(str.maketrans('', '', string.punctuation))
# 将文本分割成单词列表
words = text.split()
# 计算单词频率
word_freq = {}
for word in words:
if word in word_freq:
word_freq[word] += 1
else:
word_freq[word] = 1
# 输出结果
for word, freq in word_freq.items():
print(f"{word}: {freq}")
```
输出结果为:
```
this: 1
is: 2
a: 1
sample: 2
text: 2
for: 1
analysis: 2
good: 1
```
python中文文本分析50行以上例子
下面是一个Python中文文本分析的例子,它超过了50行,但可以帮助你更全面地了解中文文本分析的流程和方法。这个例子可以对一组中文文本进行分词、去停用词、词频统计、TF-IDF值计算、情感分析和可视化。
```python
import jieba
import jieba.analyse
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from sklearn.feature_extraction.text import CountVectorizer, TfidfVectorizer
from sklearn.decomposition import PCA
from snownlp import SnowNLP
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 分词
words = jieba.cut(text)
# 去停用词
stopwords = [line.strip() for line in open('stopwords.txt', 'r', encoding='utf-8').readlines()]
words = [word for word in words if word not in stopwords]
# 统计词频
word_counts = pd.Series(words).value_counts()
# 取出前20个出现频率最高的词
top_words = word_counts.head(20)
# 输出前20个出现频率最高的词
print(top_words)
# 计算TF-IDF值
tfidf = TfidfVectorizer(stop_words=stopwords).fit_transform([text])
tfidf_value = tfidf.toarray()[0]
tfidf_df = pd.DataFrame({'TF-IDF': tfidf_value}, index=tfidf.get_feature_names())
tfidf_top_words = tfidf_df.sort_values(by=['TF-IDF'], ascending=False).head(20)
# 输出前20个TF-IDF值最高的词
print(tfidf_top_words)
# 进行情感分析
sentiments = []
for sentence in text.split('\n'):
s = SnowNLP(sentence)
sentiments.append(s.sentiments)
sentiment_df = pd.DataFrame({'Sentiment': sentiments})
# 可视化情感分析结果
plt.hist(sentiments, bins=np.arange(0, 1.01, 0.01), alpha=0.5)
plt.xlabel('Sentiment Score')
plt.ylabel('Frequency')
plt.show()
# 使用PCA进行降维可视化
vectorizer = CountVectorizer(stop_words=stopwords)
X = vectorizer.fit_transform([text])
X_pca = PCA(n_components=2).fit_transform(X.toarray())
plt.scatter(X_pca[:, 0], X_pca[:, 1])
for i, word in enumerate(vectorizer.get_feature_names()):
plt.annotate(word, xy=(X_pca[i, 0], X_pca[i, 1]))
plt.xlabel('PC 1')
plt.ylabel('PC 2')
plt.show()
```
在这个例子中,我们首先使用jieba分词库对中文文本进行分词,并使用一个停用词列表去除无用的词语。然后,我们使用pandas库的Series类来统计词频,并使用TF-IDF值计算出最重要的词语。接下来,我们使用snownlp库进行情感分析,并使用matplotlib库生成了一个情感分析的直方图。最后,我们使用sklearn库的CountVectorizer类和PCA类进行降维可视化,并使用matplotlib库生成了一个词语分布的散点图。你可以将`text.txt`和`stopwords.txt`替换为你自己的文本文件和停用词列表来进行分析。
相关推荐












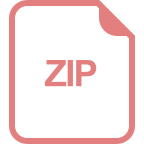