Unity c#循环列表
时间: 2024-02-05 21:05:05 浏览: 25
在 Unity C# 中,你可以使用 List<T> 类型创建一个循环列表。下面是一个示例代码:
```csharp
using System.Collections.Generic;
using UnityEngine;
public class CircularList<T>
{
private List<T> list;
private int currentIndex = 0;
public CircularList(List<T> list)
{
this.list = list;
}
public T Next()
{
if (currentIndex == list.Count - 1)
{
currentIndex = 0;
}
else
{
currentIndex++;
}
return list[currentIndex];
}
public T Previous()
{
if (currentIndex == 0)
{
currentIndex = list.Count - 1;
}
else
{
currentIndex--;
}
return list[currentIndex];
}
public T Current()
{
return list[currentIndex];
}
public void SetCurrentIndex(int index)
{
currentIndex = index;
}
}
```
这个类接受一个 List<T> 对象作为参数,并提供了三个方法:Next(), Previous() 和 Current()。Next() 方法返回下一个元素,Previous() 方法返回上一个元素,Current() 方法返回当前元素。这些方法通过维护一个 currentIndex 变量来跟踪当前元素的索引。如果当前索引到达列表的末尾或开头,它将循环回到另一端。
你可以使用这个类来创建一个循环列表并遍历它的元素,例如:
```csharp
using System.Collections.Generic;
using UnityEngine;
public class Example : MonoBehaviour
{
public List<string> items = new List<string>() { "A", "B", "C", "D" };
private CircularList<string> circularList;
void Start()
{
circularList = new CircularList<string>(items);
Debug.Log(circularList.Current()); // 输出 "A"
Debug.Log(circularList.Next()); // 输出 "B"
Debug.Log(circularList.Next()); // 输出 "C"
Debug.Log(circularList.Previous()); // 输出 "B"
circularList.SetCurrentIndex(3);
Debug.Log(circularList.Current()); // 输出 "D"
}
}
```
这个例子创建了一个字符串列表并将其传递给 CircularList<T> 类。然后,它使用 CircularList<T> 的方法来遍历列表,并输出每个元素的值。你可以使用 SetCurrentIndex() 方法来设置当前元素的索引。
相关推荐
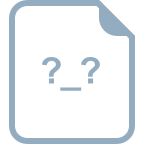














