写一个获取excel表格数据的详细java代码
时间: 2024-09-07 08:06:04 浏览: 66
获取Excel表格数据通常涉及到使用第三方库,比如Apache POI。Apache POI是一个Java库,可以用来读取和写入Microsoft Office格式的文件,包括Excel。以下是使用Apache POI来读取Excel文件数据的简单示例:
首先,你需要添加Apache POI依赖到你的项目中。如果你使用Maven,可以在`pom.xml`文件中添加以下依赖:
```xml
<dependencies>
<!-- Apache POI dependency for Excel -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.2.3</version>
</dependency>
</dependencies>
```
然后,你可以使用以下Java代码来读取Excel文件中的数据:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileInputStream;
import java.util.Iterator;
public class ExcelReader {
public static void main(String[] args) {
String excelFilePath = "example.xlsx"; // 指定Excel文件路径
FileInputStream fis = null;
Workbook workbook = null;
Sheet sheet = null;
try {
fis = new FileInputStream(new File(excelFilePath));
workbook = new XSSFWorkbook(fis);
sheet = workbook.getSheetAt(0); // 获取第一个工作表
Iterator<Row> rowIterator = sheet.iterator();
while (rowIterator.hasNext()) {
Row row = rowIterator.next();
Iterator<Cell> cellIterator = row.cellIterator();
while (cellIterator.hasNext()) {
Cell cell = cellIterator.next();
switch (cell.getCellType()) {
case STRING:
System.out.print(cell.getStringCellValue() + "\t");
break;
case NUMERIC:
System.out.print(cell.getNumericCellValue() + "\t");
break;
case BOOLEAN:
System.out.print(cell.getBooleanCellValue() + "\t");
break;
case FORMULA:
System.out.print(cell.getCellFormula() + "\t");
break;
default:
System.out.print(" " + "\t");
}
}
System.out.println();
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (workbook != null) {
workbook.close();
}
if (fis != null) {
fis.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
```
这段代码将打开一个名为`example.xlsx`的Excel文件,并读取第一个工作表中的所有单元格数据。它会检查每个单元格的类型,并根据类型输出相应的数据。
阅读全文
相关推荐
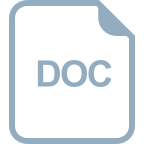
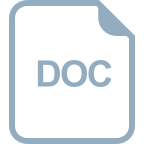
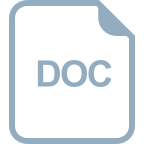
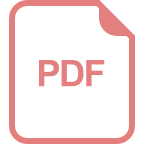







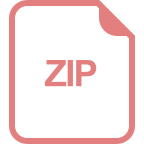
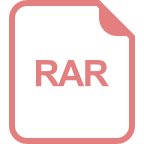
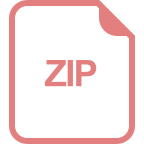
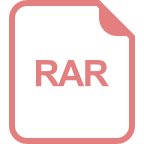
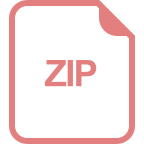


