@RequestParam怎么校验非空
时间: 2023-11-14 19:10:22 浏览: 38
可以使用@RequestParam注解的required属性来校验非空。将required属性设置为true,如果请求参数中没有该参数或者该参数的值为null,则会抛出MissingServletRequestParameterException异常。
例如:
```
@GetMapping("/test")
public String test(@RequestParam(name = "name", required = true) String name) {
return "Hello " + name;
}
```
如果请求中没有name参数或者name参数的值为null,就会抛出MissingServletRequestParameterException异常。
相关问题
@RequestParam @NotNull
@RequestParam 和 @NotNull 是两个不同的注解,通常用于后端开发中的参数传递和参数校验。
@RequestParam 是 Spring 框架中的一个注解,用于从请求中获取参数的值。当我们在后端接口中使用 @RequestParam 注解时,Spring 会根据参数名从请求中获取对应的值并赋给方法参数。
例如,假设有一个 GET 请求 /api/user,请求参数为 username,那么我们可以在后端接口中这样使用 @RequestParam 注解来获取该参数的值:
```java
@GetMapping("/api/user")
public ResponseEntity<User> getUserByUsername(@RequestParam("username") String username) {
// 根据用户名查询用户信息
// ...
}
```
@NotNull 是 Java 校验框架中的一个注解,用于校验方法参数或字段是否为空。当我们在后端接口中使用 @NotNull 注解时,框架会在执行方法前进行校验,如果方法参数或字段为 null,则会抛出异常。
例如,假设有一个后端接口 createUser,需要传入一个非空的用户名,那么我们可以在方法参数上使用 @NotNull 注解进行校验:
```java
@PostMapping("/api/user")
public ResponseEntity<String> createUser(@NotNull @RequestParam("username") String username) {
// 创建用户
// ...
}
```
这样,在调用 createUser 接口时,如果未传入用户名或用户名为空,则会抛出异常。
总结:
@RequestParam 用于从请求中获取参数的值,而 @NotNull 用于校验方法参数或字段是否为空。它们在后端开发中常用于参数传递和参数校验。
@Override public boolean findUserInfoByIdAndName(@RequestParam Integer name, @RequestParam String age) throws Exception { if (name == null) { throw new Exception("用户名不能为空"); } if (age == null) { throw new Exception("年龄不能为空"); } String ageStr = "18"; if (age < 120) { return true; } throw new Exception("年龄必须大于18且小于120"); UserInfo userInfo = deviceInfoMapper.findUserInfoByIdAndName(name,age); if (userInfo == null) { throw new Exception("未找到符合条件的用户信息"); }
这段代码是一个Java方法,它的作用是根据用户名和年龄查询用户信息。方法的参数包括一个整型的name和一个字符串类型的age,返回一个布尔类型的值。
在方法中,首先会进行参数的非空校验,如果参数为空,则抛出异常。然后会对年龄进行判断,如果年龄小于18或大于120,则抛出异常。
接下来会调用一个名为findUserInfoByIdAndName的方法,该方法会根据传入的用户名和年龄进行查询用户信息的操作。如果查询结果为null,则表示未找到符合条件的用户信息,会抛出异常。
最后,如果整个方法执行成功,会返回true。
相关推荐
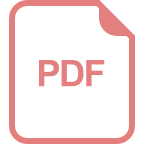
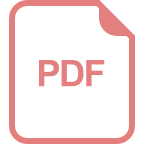







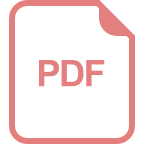
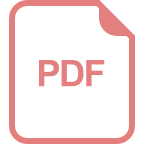
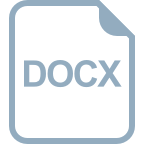
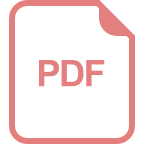
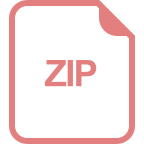