python matplotlib example
时间: 2023-10-12 20:09:30 浏览: 38
Here is an example of how to use matplotlib in Python to plot a simple line graph:
```python
import matplotlib.pyplot as plt
# Create some sample data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Create the plot
plt.plot(x, y)
# Add labels and title
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Sample Line Graph')
# Show the plot
plt.show()
```
This code will produce a simple line graph with x-values 1 to 5 on the x-axis and y-values 2 to 10 on the y-axis. The plot will have labels for the x and y axes and a title at the top. The `plt.show()` function is used to display the plot.
相关问题
python matplotlib wait
In Python, the `matplotlib` library does not have a built-in "wait" function. However, you can use the `plt.show()` function to display the plot and block the execution of the program until the plot window is closed by the user. Here's an example:
```python
import matplotlib.pyplot as plt
# Plotting code here
plt.show() # Display the plot and wait for user interaction
print("This line will be executed after the plot window is closed.")
```
After calling `plt.show()`, the program will pause and wait for the user to close the plot window. Once the plot window is closed, the program will continue executing the remaining code.
pythonmatplotlib保存图片
要保存 matplotlib 绘制的图片,可以使用 `savefig()` 函数。该函数可以将图片保存为多种格式,如 PNG、PDF、SVG 等。以下是保存为 PNG 格式的示例代码:
```python
import matplotlib.pyplot as plt
# 绘制图形
fig, ax = plt.subplots()
ax.plot([1, 2, 3], [4, 5, 6])
# 保存为 PNG 格式
fig.savefig('example.png')
```
这段代码会生成一张包含一条折线的图形,并将该图形保存为名为 `example.png` 的 PNG 图片。你可以将文件名更改为其他格式,以保存为其他类型的图片。
相关推荐
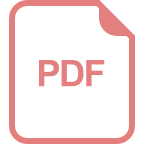
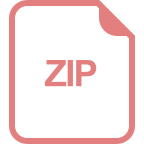
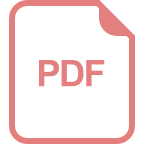












