c++怎麽在agv調度系統中,怎麽去用任務觸發agv做任務,直到任務全部做完
时间: 2024-03-03 11:46:49 浏览: 51
在 C++ 中实现 AGV 调度系统,可以使用多线程实现任务触发 AGV 执行。具体实现步骤如下:
1. 定义 AGV 类
首先需要定义一个 AGV 类,包含 AGV 的属性和方法,如 AGV 编号、当前任务、执行任务的方法等。
```c++
class AGV {
public:
// 构造函数,传入 AGV 编号
AGV(int id);
// 执行任务的方法,传入任务编号
void executeTask(int task_id);
// 获取 AGV 编号
int getId();
private:
int id_; // AGV 编号
int current_task_id_; // 当前任务编号
std::mutex mutex_; // 互斥锁,保证线程安全
};
```
2. 定义任务类
定义一个任务类,包含任务属性和方法,如任务编号、任务执行时间、任务执行方法等。
```c++
class Task {
public:
// 构造函数,传入任务编号和执行时间
Task(int id, std::chrono::system_clock::time_point time_point);
// 执行任务的方法,传入 AGV 对象
void execute(AGV& agv);
// 获取任务编号
int getId();
// 获取任务执行时间
std::chrono::system_clock::time_point getTimePoint();
private:
int id_; // 任务编号
std::chrono::system_clock::time_point time_point_; // 任务执行时间
};
```
3. 实现任务触发
创建一个任务队列,存储所有的任务。创建多个 AGV 对象,每个 AGV 对象都是一个线程,不断地从任务队列中获取任务,如果任务的执行时间已经到了,就执行该任务。如果任务队列为空,就等待新的任务加入。
```c++
#include <thread>
#include <queue>
int main()
{
// 创建任务队列,存储所有的任务
std::priority_queue<Task> task_queue;
// 创建多个 AGV 对象,每个 AGV 对象都是一个线程
std::vector<std::thread> agv_threads;
for (int i = 0; i < num_of_agvs; ++i) {
AGV agv(i);
agv_threads.emplace_back([&]() {
while (true) {
// 获取任务队列中的任务
std::lock_guard<std::mutex> lock(mutex_);
if (task_queue.empty()) {
// 如果任务队列为空,就等待新的任务加入
continue;
}
Task task = task_queue.top();
if (std::chrono::system_clock::now() >= task.getTimePoint()) {
// 如果任务的执行时间已经到了,就执行该任务
task.execute(agv);
task_queue.pop();
}
}
});
}
// 等待所有 AGV 对象执行完所有任务
for (auto& thread : agv_threads) {
thread.join();
}
return 0;
}
```
4. 实现任务执行
在任务的执行方法中,先判断 AGV 是否正在执行其他任务,如果是,就等待其他任务执行完毕。如果 AGV 没有在执行其他任务,就执行当前任务。
```c++
void Task::execute(AGV& agv)
{
// 判断 AGV 是否正在执行其他任务
std::lock_guard<std::mutex> lock(agv.mutex_);
while (agv.current_task_id_ != -1) {
// 如果 AGV 正在执行其他任务,就等待
std::this_thread::sleep_for(std::chrono::milliseconds(100));
}
// 如果 AGV 没有在执行其他任务,就执行当前任务
agv.current_task_id_ = id_;
agv.executeTask(id_);
agv.current_task_id_ = -1;
}
```
这样,就可以实现任务触发 AGV 执行,直到所有任务全部执行完毕。
阅读全文
相关推荐
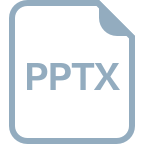
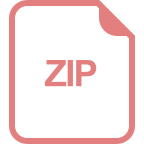
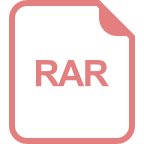
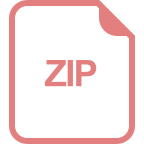
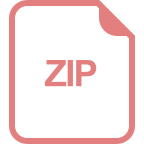
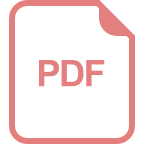
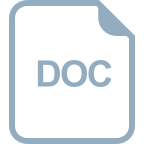
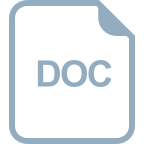
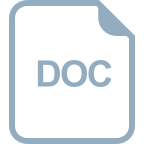
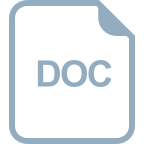
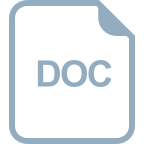


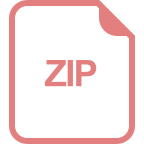
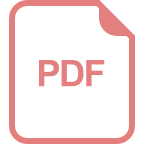