vue3.0修改用户功能
时间: 2023-11-03 18:04:42 浏览: 45
要修改用户功能,你需要先确定你的 Vue 3.0 应用是如何获取和展示用户数据的。通常情况下,你需要创建一个 Vue 组件来展示用户数据,然后在该组件中添加修改用户信息的功能。
以下是一个示例:
1. 创建一个展示用户信息的组件,例如 "User" 组件。
2. 在该组件中,使用 Vue 的数据绑定功能来展示用户信息。
3. 创建一个表单来收集用户修改后的信息。
4. 添加一个 "修改" 按钮,当用户点击该按钮时,提交表单中的数据,并发送一个更新用户信息的请求到后端。
5. 在组件中处理请求的响应,并更新组件中展示的用户信息。
下面是一个简单的示例代码,假设你的后端接口是 "/api/user",你需要使用 axios 库来发送请求:
```
<template>
<div>
<h1>{{ user.username }}</h1>
<p>{{ user.email }}</p>
<form @submit.prevent="updateUser">
<input type="text" v-model="formData.username" />
<input type="email" v-model="formData.email" />
<button type="submit">修改</button>
</form>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
user: {},
formData: {
username: '',
email: ''
}
};
},
mounted() {
// 获取用户信息并展示
axios.get('/api/user')
.then(response => {
this.user = response.data;
this.formData.username = response.data.username;
this.formData.email = response.data.email;
})
.catch(error => {
console.log(error);
});
},
methods: {
updateUser() {
// 发送更新用户信息请求
axios.put('/api/user', this.formData)
.then(response => {
this.user = response.data;
this.formData.username = response.data.username;
this.formData.email = response.data.email;
})
.catch(error => {
console.log(error);
});
}
}
};
</script>
```
这只是一个简单的示例,实际应用中可能需要添加更多的逻辑和验证。同时,你还需要确保后端接口能够正确地处理更新用户信息的请求。
相关推荐
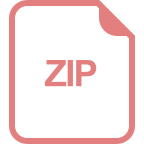
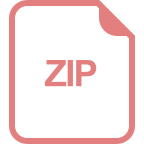
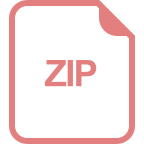
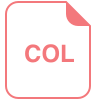
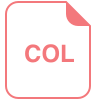
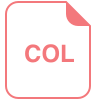
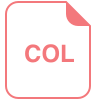
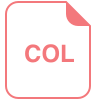









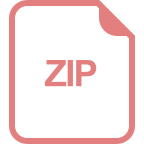