Graphics2D 画图图片
时间: 2023-10-23 08:11:03 浏览: 98
Graphics2D 是 Java 2D API 中的一个类,用于在 Java 应用程序中绘制 2D 图形。Graphics2D 类提供了一些方法来处理不同类型的 2D 图形,例如线条、矩形、椭圆、弧形、多边形等。
要使用 Graphics2D 类来绘制图片,可以使用以下步骤:
1. 创建一个 BufferedImage 对象,该对象可以用来存储要绘制的图片。
```
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);
```
其中,width 和 height 分别是图片的宽度和高度,BufferedImage.TYPE_INT_ARGB 是指图像类型为 32 位 ARGB。
2. 获取 Graphics2D 对象,该对象可以用来绘制图形。
```
Graphics2D g2d = image.createGraphics();
```
3. 使用 Graphics2D 对象的 drawImage() 方法将所需的图像绘制到 BufferedImage 对象上。
```
g2d.drawImage(img, x, y, null);
```
其中,img 是要绘制的图像,x 和 y 分别是图像的起始位置的 x 和 y 坐标。
4. 最后,关闭 Graphics2D 对象。
```
g2d.dispose();
```
完整的代码示例:
```java
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
public class ImageDraw {
public static void main(String[] args) {
int width = 500;
int height = 500;
// 创建 BufferedImage 对象
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);
// 获取 Graphics2D 对象
Graphics2D g2d = image.createGraphics();
// 绘制图像
try {
BufferedImage img = ImageIO.read(new File("image.jpg"));
int x = (width - img.getWidth()) / 2;
int y = (height - img.getHeight()) / 2;
g2d.drawImage(img, x, y, null);
} catch (Exception e) {
e.printStackTrace();
}
// 关闭 Graphics2D 对象
g2d.dispose();
// 保存图像到文件
try {
ImageIO.write(image, "png", new File("output.png"));
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
上述代码将会创建一个 500x500 像素的 BufferedImage 对象,并将一张名为 "image.jpg" 的图像绘制在中心位置,最后将结果保存为名为 "output.png" 的 PNG 图像文件。
阅读全文
相关推荐
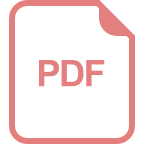

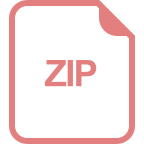
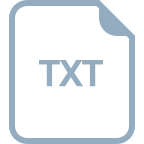
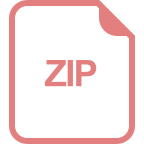
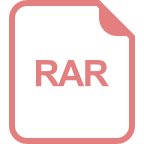
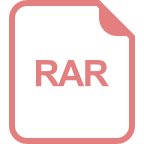
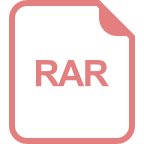
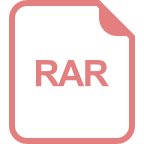
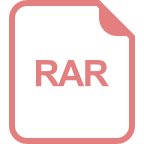
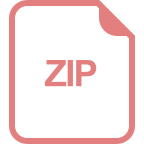
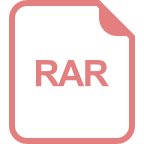
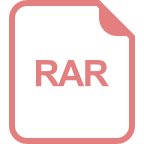
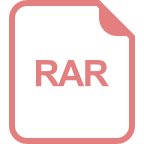
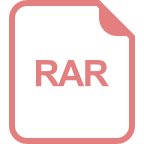
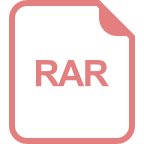
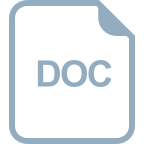