pyqt5设置按钮点击后抖动效果
时间: 2023-08-16 16:05:14 浏览: 55
要实现按钮点击后的抖动效果,可以使用QPropertyAnimation类和QParallelAnimationGroup类。
以下是一个简单的示例代码:
```python
from PyQt5.QtCore import QPropertyAnimation, QEasingCurve, QRect
from PyQt5.QtGui import QPalette, QColor
from PyQt5.QtWidgets import QApplication, QPushButton, QWidget, QParallelAnimationGroup
class MyButton(QPushButton):
def __init__(self, *args, **kwargs):
super(MyButton, self).__init__(*args, **kwargs)
self.animation_group = QParallelAnimationGroup(self)
self.hover_animation = QPropertyAnimation(self, b"color")
self.click_animation = QPropertyAnimation(self, b"geometry")
self.hover_animation.setStartValue(QColor(0, 0, 0))
self.hover_animation.setEndValue(QColor(255, 255, 255))
self.hover_animation.setDuration(300)
self.hover_animation.setEasingCurve(QEasingCurve.InOutCirc)
self.click_animation.setDuration(50)
self.click_animation.setEasingCurve(QEasingCurve.InOutCirc)
self.animation_group.addAnimation(self.hover_animation)
self.animation_group.addAnimation(self.click_animation)
self.clicked.connect(self.animate)
def enterEvent(self, event):
self.hover_animation.setDirection(QPropertyAnimation.Forward)
self.animation_group.start()
def leaveEvent(self, event):
self.hover_animation.setDirection(QPropertyAnimation.Backward)
self.animation_group.start()
def animate(self):
self.click_animation.setStartValue(self.geometry())
self.click_animation.setEndValue(self.geometry().adjusted(2, 2, -2, -2))
self.click_animation.start()
class MainWindow(QWidget):
def __init__(self):
super(MainWindow, self).__init__()
self.button = MyButton("Click me", self)
self.button.setGeometry(50, 50, 100, 50)
self.show()
if __name__ == "__main__":
app = QApplication([])
palette = QPalette()
palette.setColor(QPalette.Button, QColor(255, 0, 0))
app.setPalette(palette)
window = MainWindow()
app.exec_()
```
在这个示例中,我们自定义了一个MyButton类,它继承自QPushButton类,并实现了enterEvent、leaveEvent和animate方法。当鼠标移入按钮时,我们会启动一个颜色渐变动画;当鼠标移出按钮时,我们会再次启动这个动画,但是方向相反。当按钮被点击时,我们会启动一个位置动画,让按钮的位置稍微抖动一下,以达到抖动的效果。
注意,这个示例代码使用了QPalette来设置应用程序的主题色。如果你想使用不同的颜色,可以自行修改。
相关推荐
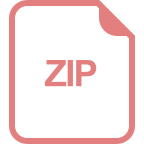
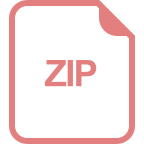
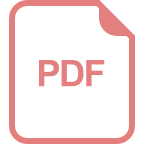














