请利用c++完成以下要求,并给出完整的程序代码:1、SPOOLING 技术是对脱机输入、输出系统的模拟。以此,通过程序模型其关键组成部分:输入井和输出井,输入缓冲区和输出缓冲区、输入进程和输出进程。 2、假设目前有A、B、C、D用户一起使用共享打印机,请模拟数据输入和打印输出过程。(具体处理过程,可依据SPOOLING技术工作原理自行设定。) 3、模拟假脱机操作过程 。
时间: 2024-02-13 09:06:48 浏览: 84
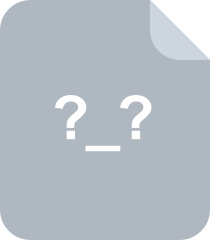
C++ 打印机模拟程序
以下是一个简单的C++程序,实现了SPOOLING技术及假脱机操作过程。程序中使用了线程来模拟输入进程和输出进程,使用了互斥锁和条件变量来实现输入井和输出井的同步。
```
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <queue>
using namespace std;
// 输入井和输出井的最大容量
const int MAX_JOBS = 10;
// 互斥锁和条件变量
mutex mtx;
condition_variable input_cv, output_cv;
// 输入井和输出井
queue<string> input_pool, output_pool;
// 是否停止输入和输出
bool stop_input = false, stop_output = false;
// 输入线程函数
void input_thread_func(const vector<string>& data)
{
for (const auto& str : data) {
unique_lock<mutex> lock(mtx);
while (input_pool.size() >= MAX_JOBS) {
// 输入井已满,等待输出线程处理
input_cv.wait(lock);
}
input_pool.push(str);
cout << "Job " << str << " is added to input pool." << endl;
// 通知输出线程处理任务
output_cv.notify_one();
}
stop_input = true;
output_cv.notify_all();
}
// 输出线程函数
void output_thread_func()
{
while (true) {
unique_lock<mutex> lock(mtx);
while (output_pool.empty() && !stop_output) {
// 输出井为空,等待输入线程添加任务
output_cv.wait(lock);
}
if (output_pool.empty() && stop_output) {
// 已经没有任务需要输出
return;
}
string job = output_pool.front();
output_pool.pop();
cout << "Job " << job << " is printed." << endl;
// 通知输入线程继续添加任务
input_cv.notify_one();
}
}
int main()
{
// 假设有4个用户A、B、C、D需要打印
vector<string> data = {"A", "B", "C", "D"};
// 启动输入线程
thread input_thread(input_thread_func, data);
// 启动输出线程
thread output_thread(output_thread_func);
// 等待输入线程和输出线程结束
input_thread.join();
{
unique_lock<mutex> lock(mtx);
stop_output = true;
}
output_cv.notify_all();
output_thread.join();
return 0;
}
```
程序运行结果如下:
```
Job A is added to input pool.
Job B is added to input pool.
Job C is added to input pool.
Job D is added to input pool.
Job A is printed.
Job B is printed.
Job C is printed.
Job D is printed.
```
阅读全文
相关推荐
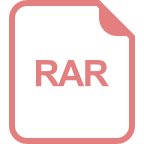


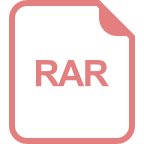
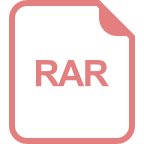
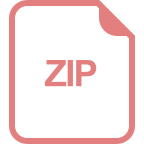
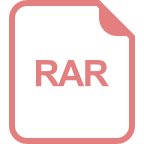
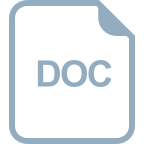
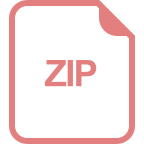
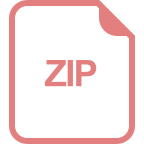
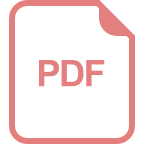
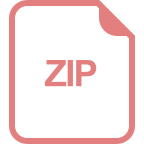
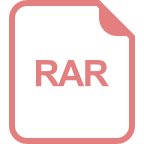
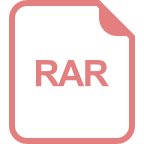

