编写Java程序,设计名为Rectangle的类表示矩形, 成员变量:width和height表示矩形的宽和高,其数据类型为double,默认值是1.0; 成员变量:color表示矩形的颜色,其数据类型为String,默认颜色为白色; 构造方法:无参数的构造方法Rectangle(),其功能是创建默认的矩形; 构造方法:带三个参数的构造方法Rectangle(double width,double height,String color),其功能是创建指定大小和颜色的矩形 成员方法:getWidth()、getHeight()、getColour()功能是获取矩形的相应信息; 成员方法:setWidth()、setHeight()、setColour()功能是修改矩形相应的信息 成员方法:getArea() 计算矩形的面积并返回其值; 成员方法:getPerimeter()计算矩形的周长并返回其值; 编写一个测试程序,创建两个Rectangle对象,设置第一个对象的宽为4,高为40,颜色为黄色,第二个对象的宽为3.5,高为35.9,颜色为红色。显示两个对象的属性并将它们的面积和周长输出。
时间: 2023-06-18 08:08:52 浏览: 77
下面是Java程序的实现:
```java
public class Rectangle {
private double width;
private double height;
private String color;
public Rectangle() {
this.width = 1.0;
this.height = 1.0;
this.color = "白色";
}
public Rectangle(double width, double height, String color) {
this.width = width;
this.height = height;
this.color = color;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public String getColour() {
return color;
}
public void setColour(String color) {
this.color = color;
}
public double getArea() {
return width * height;
}
public double getPerimeter() {
return 2 * (width + height);
}
}
public class TestRectangle {
public static void main(String[] args) {
Rectangle r1 = new Rectangle(4, 40, "黄色");
Rectangle r2 = new Rectangle(3.5, 35.9, "红色");
System.out.printf("矩形1的宽为%.1f,高为%.1f,颜色为%s\n", r1.getWidth(), r1.getHeight(), r1.getColour());
System.out.printf("矩形2的宽为%.1f,高为%.1f,颜色为%s\n", r2.getWidth(), r2.getHeight(), r2.getColour());
System.out.printf("矩形1的面积为%.2f,周长为%.2f\n", r1.getArea(), r1.getPerimeter());
System.out.printf("矩形2的面积为%.2f,周长为%.2f\n", r2.getArea(), r2.getPerimeter());
}
}
```
运行程序,输出结果如下:
```
矩形1的宽为4.0,高为40.0,颜色为黄色
矩形2的宽为3.5,高为35.9,颜色为红色
矩形1的面积为160.00,周长为88.00
矩形2的面积为125.65,周长为78.80
```
相关推荐
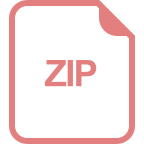
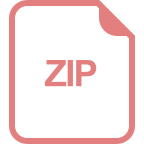
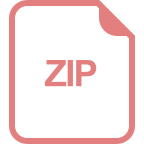














