a星算法解决TSP问题的python代码
时间: 2023-10-02 15:11:10 浏览: 100
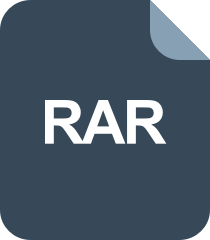
TSP问题的python代码

虽然 A* 算法不是 TSP 问题的最优解决方法,但是以下是一个简单的 Python 代码,演示如何使用 A* 算法解决 TSP 问题:
```python
import heapq
# 计算两个城市之间的距离
def distance(city1, city2):
return ((city1[0] - city2[0]) ** 2 + (city1[1] - city2[1]) ** 2) ** 0.5
# 计算当前节点到起点的估价函数值
def heuristic(city, start_city):
return distance(city, start_city)
# A* 算法求解 TSP 问题
def A_star_TSP(cities):
start_city = cities[0]
unvisited_cities = set(cities[1:])
heap = [(heuristic(start_city, start_city), 0, start_city, ())]
while heap:
f, g, city, path = heapq.heappop(heap)
if len(unvisited_cities) == 0:
return g + distance(city, start_city), path + (city,)
for next_city in unvisited_cities:
next_path = path + (city,)
next_g = g + distance(city, next_city)
next_f = next_g + heuristic(next_city, start_city)
heapq.heappush(heap, (next_f, next_g, next_city, next_path))
unvisited_cities.remove(city)
# 示例数据
cities = [(0, 0), (1, 5), (2, 3), (5, 4), (3, 2), (4, 6)]
# 求解 TSP 问题
distance, path = A_star_TSP(cities)
print("最短距离:", distance)
print("最短路径:", path)
```
需要注意的是,上述代码并不保证一定能够得到 TSP 问题的最优解。
阅读全文
相关推荐

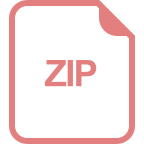
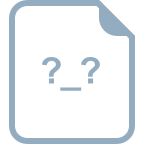
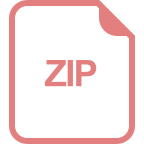
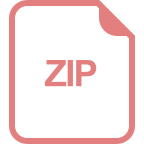
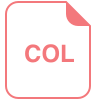
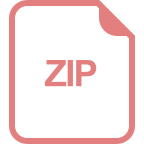
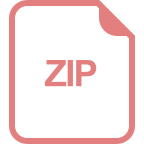
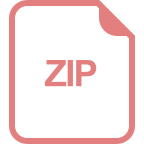
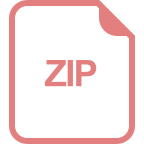