QVariant QMySQlQueryModel::data(const QModelIndex &item, int role) const
时间: 2024-06-01 08:08:31 浏览: 11
这是一个函数定义,用于 QMySQlQueryModel 类的实现。它是 Qt 框架中 QSqlQueryModel 类的子类,用于连接 MySQL 数据库并执行查询操作。
QVariant QMySQlQueryModel::data(const QModelIndex &item, int role) const 的作用是返回指定索引处的数据。其中,item 是要返回数据的模型索引,role 是要返回的数据的角色。
该函数的返回值是 QVariant 类型,可以存储任何类型的数据。在使用时,可以根据 role 参数的不同返回不同的数据,例如返回单元格的文本、颜色等。
需要注意的是,该函数是一个虚函数,可以在子类中进行重写以实现特定的功能。
相关问题
QVariant Tasklist::data(const QModelIndex &index, int role) const { int row = index.row(); if(row < 0 || row >= data_list.count()) { return QVariant(); } const Data &data = data_list[row]; switch (role) { case TitleRole: return data.title_; case produ_modle_role: return data.product_modle; case assembly_no_role: return data.assembly_no; case bach_no_role: return data.bach_no; case scheduled_finish_time_role: return data.scheduled_finish_time; default: return QVariant(); } }
这段代码是一个`Tasklist`类的实现中的一个函数,用于返回一个指定索引的数据。这个函数的具体作用是:
1. 获取指定索引对应的行数`row`。
2. 如果`row`小于0或者大于等于`data_list`列表的元素个数,则返回一个空的QVariant。
3. 否则,获取`data_list`列表中指定行数`row`对应的数据`data`。
4. 根据指定的`role`参数,返回对应的数据。这里,`role`是一个自定义的枚举值,表示要返回的数据的类型。如果`role`不是已定义的枚举值,则返回一个空的QVariant。
具体来说,这个函数是在实现Qt中的Model/View框架中的一个接口函数`QAbstractItemModel::data()`,用于在View中显示Model中的数据。在这个函数中,我们可以根据需要,自定义返回数据的类型和格式。在这个例子中,我们定义了几个自定义的枚举值,分别表示要返回的几种数据类型,然后根据传入的`role`参数,返回对应的数据。
c++实现qtableview撤销回退setData(const QModelIndex &index, const QVariant &value, int role = Qt::EditRole)
要实现QTableView的撤销/回退功能,需要记录下每次setData的修改前和修改后的数据,以便撤销时能够恢复原来的数据。
具体实现可以创建一个自定义的QStandardItemModel子类,重写setData方法,记录下修改前和修改后的数据,同时还要记录下操作的行列信息。在撤销时,将修改后的数据恢复为修改前的数据即可。
以下是一个简单的示例代码:
```c++
class MyTableModel : public QStandardItemModel
{
public:
explicit MyTableModel(int rows, int columns, QObject* parent = nullptr)
: QStandardItemModel(rows, columns, parent)
{
// 初始化撤销栈
m_undoStack = new QUndoStack(this);
}
// 重写setData方法
bool setData(const QModelIndex& index, const QVariant& value, int role = Qt::EditRole) override
{
// 记录修改前的数据
QStandardItem* item = itemFromIndex(index);
QVariant oldValue = item->data(role);
// 调用基类setData方法进行修改
bool result = QStandardItemModel::setData(index, value, role);
if (result)
{
// 记录修改后的数据和操作信息
QVariant newValue = item->data(role);
QPair<QModelIndex, QVariant> edit(index, newValue);
m_edits.push(edit);
m_currentEdit = edit;
emit dataChanged(index, index);
// 将操作添加到撤销栈中
m_undoStack->push(new EditCommand(this, oldValue, newValue, index, role));
}
return result;
}
// 撤销操作
void undo()
{
if (m_undoStack->canUndo())
{
m_undoStack->undo();
if (!m_edits.isEmpty())
{
m_currentEdit = m_edits.pop();
emit dataChanged(m_currentEdit.first, m_currentEdit.first);
}
}
}
// 回退操作
void redo()
{
if (m_undoStack->canRedo())
{
m_undoStack->redo();
if (!m_edits.isEmpty())
{
m_currentEdit = m_edits.pop();
emit dataChanged(m_currentEdit.first, m_currentEdit.first);
}
}
}
private:
// 记录修改前和修改后的数据
QStack<QPair<QModelIndex, QVariant>> m_edits;
QPair<QModelIndex, QVariant> m_currentEdit;
// 撤销栈
QUndoStack* m_undoStack;
// 定义撤销命令
class EditCommand : public QUndoCommand
{
public:
EditCommand(MyTableModel* model, QVariant oldValue, QVariant newValue, QModelIndex index, int role)
: m_model(model)
, m_oldValue(oldValue)
, m_newValue(newValue)
, m_index(index)
, m_role(role)
{}
void undo()
{
m_model->setData(m_index, m_oldValue, m_role);
}
void redo()
{
m_model->setData(m_index, m_newValue, m_role);
}
private:
MyTableModel* m_model;
QVariant m_oldValue;
QVariant m_newValue;
QModelIndex m_index;
int m_role;
};
};
```
在使用时,可以将QTableView的model设置为MyTableModel的实例,并在需要撤销/回退时调用undo和redo方法即可。
相关推荐
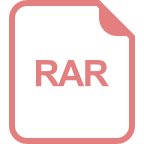
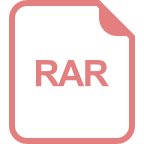













