Pthreads矩阵-向量程序C语言
时间: 2023-12-31 22:07:09 浏览: 125
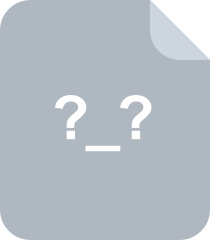
C语言矩阵程序
以下是一个使用Pthreads实现的矩阵-向量程序的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 4
#define MATRIX_SIZE 1000
int matrix[MATRIX_SIZE][MATRIX_SIZE];
int vector[MATRIX_SIZE];
int result[MATRIX_SIZE];
int chunk_size;
void *matrix_vector_multiply(void *thread_id) {
int tid = *(int*)thread_id;
int start = tid * chunk_size;
int end = start + chunk_size;
for (int i = start; i < end; i++) {
int sum = 0;
for (int j = 0; j < MATRIX_SIZE; j++) {
sum += matrix[i][j] * vector[j];
}
result[i] = sum;
}
pthread_exit(NULL);
}
int main() {
pthread_t threads[NUM_THREADS];
int thread_ids[NUM_THREADS];
// Initialize matrix and vector
for (int i = 0; i < MATRIX_SIZE; i++) {
for (int j = 0; j < MATRIX_SIZE; j++) {
matrix[i][j] = rand() % 10;
}
vector[i] = rand() % 10;
}
// Divide the work among the threads
chunk_size = MATRIX_SIZE / NUM_THREADS;
// Create threads
for (int i = 0; i < NUM_THREADS; i++) {
thread_ids[i] = i;
pthread_create(&threads[i], NULL, matrix_vector_multiply, &thread_ids[i]);
}
// Wait for threads to finish
for (int i = 0; i < NUM_THREADS; i++) {
pthread_join(threads[i], NULL);
}
// Print result vector
printf("Result vector:\n");
for (int i = 0; i < MATRIX_SIZE; i++) {
printf("%d ", result[i]);
}
printf("\n");
return 0;
}
```
该程序将矩阵和向量初始化为随机值,然后将矩阵乘以向量,将结果存储在result数组中。程序使用pthread_create函数创建线程,并使用pthread_join函数等待线程完成。每个线程计算矩阵的一部分,以便并行执行。程序使用一个chunk_size变量来确定每个线程需要处理的矩阵的行数。
阅读全文
相关推荐

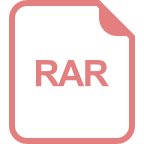
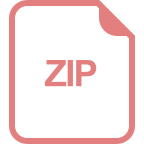
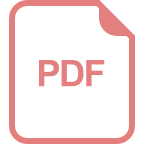
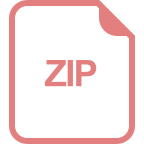
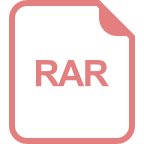
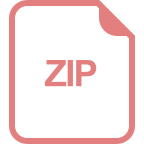
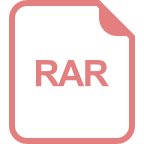
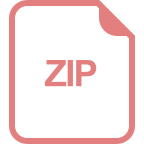
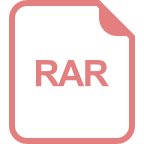


