通过IOC容器分别创建Teacher和Student对象,要求对象中有姓名、学号(或工号)、所在学院、性别等属性,并为各属性赋值。 根据bean的类型从IOC容器中获取bean的实例。 通过构造器为Teacher和Student对象赋值。通过JAVA语言实现
时间: 2024-09-21 14:13:52 浏览: 38
在Java中,可以使用Spring框架的IoC(Inversion of Control,控制反转)容器来管理和创建对象。首先,你需要定义`Teacher`和`Student`这两个类,每个类都有姓名、学号(或工号)、所在学院和性别等属性。例如:
```java
public class Teacher {
private String name;
private String studentId;
private String college;
private String gender;
// getters and setters...
public Teacher(String name, String studentId, String college, String gender) {
this.name = name;
this.studentId = studentId;
this.college = college;
this.gender = gender;
}
}
public class Student {
private String name;
private String id;
private String college;
private String gender;
// getters and setters...
public Student(String name, String id, String college, String gender) {
this.name = name;
this.id = id;
this.college = college;
this.gender = gender;
}
}
```
然后,在Spring配置文件(如applicationContext.xml或通过Java配置@Configuration注解的类)中,你可以定义bean并指定它们的类型:
```xml
<!-- XML配置 -->
<bean id="teacherBean" class="com.example.Teacher">
<constructor-arg>
<value>张三</value>
</constructor-arg>
<constructor-arg>
<value>001</value>
</constructor-arg>
<constructor-arg>
<value>计算机科学系</value>
</constructor-arg>
<constructor-arg>
<value>男</value>
</constructor-arg>
</bean>
<bean id="studentBean" class="com.example.Student">
<constructor-arg>
<value>李四</value>
</constructor-arg>
<constructor-arg>
<value>002</value>
</constructor-arg>
<constructor-arg>
<value>商学院</value>
</constructor-arg>
<constructor-arg>
<value>女</value>
</constructor-arg>
</bean>
```
或者使用Java配置:
```java
@Configuration
public class AppConfig {
@Bean
public Teacher teacher() {
return new Teacher("张三", "001", "计算机科学系", "男");
}
@Bean
public Student student() {
return new Student("李四", "002", "商学院", "女");
}
}
```
最后,通过@Autowired注解或ApplicationContext来获取并使用这些bean:
```java
@Autowired
private Teacher teacherObj;
@Autowired
private Student studentObj;
```
此时,你可以直接使用`teacherObj`和`studentObj`来访问他们的属性和方法。
相关推荐
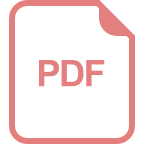
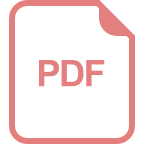

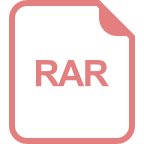
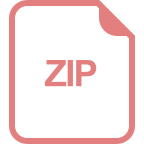
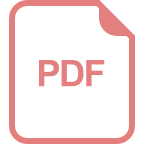
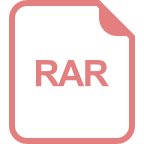
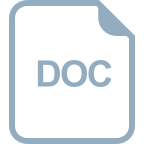
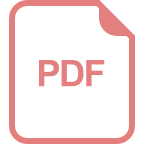
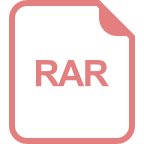
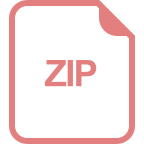
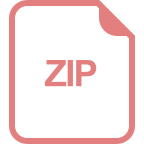