编写一个GUI程序(使用tkinter、wxpython、pyqt三个库中的一个),结合OpenCV图像处理的内容,实现对选定照片进行处理: 对照片实现几何变换:缩放(手动输入缩放宽高比)、旋转(手动输入旋转角度、翻转)
时间: 2024-11-06 12:15:25 浏览: 43
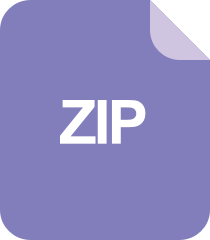
invoiceReader:使用一个兼容opencv的摄像头读取和管理发票、身份证等图像
在Python中,你可以选择使用Tkinter、wxPython或PyQt作为图形用户界面(GUI)库,配合OpenCV进行图像处理。这里以PyQt为例,因为它的QGraphicsView和QGraphicsScene组件非常适合显示和编辑图像。
首先,你需要安装必要的库,如`opencv-python`, `numpy`, 和 `PyQt5`。下面是一个基础的框架,展示了如何创建一个简单的GUI,让用户选择图片并进行几何变换操作:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QVBoxLayout, QLabel, QPushButton, QFileDialog
from PyQt5.QtGui import QPixmap, QImage, QPainter
import cv2
import numpy as np
class ImageProcessor(QMainWindow):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
self.setWindowTitle("Image Processor")
main_widget = QWidget()
layout = QVBoxLayout(main_widget)
# 图片显示部分
self.image_label = QLabel(self)
self.image_label.setFixedWidth(600)
self.image_label.setFixedHeight(400)
layout.addWidget(self.image_label)
# 按钮组
self.load_button = QPushButton("Load Image", self)
self.scale_button = QPushButton("Scale", self)
self.rotate_button = QPushButton("Rotate", self)
self.flip_button = QPushButton("Flip", self)
layout.addWidget(self.load_button)
layout.addWidget(self.scale_button)
layout.addWidget(self.rotate_button)
layout.addWidget(self.flip_button)
self.load_button.clicked.connect(self.load_image)
self.scale_button.clicked.connect(self.scale_image)
self.rotate_button.clicked.connect(self.rotate_image)
self.flip_button.clicked.connect(self.flip_image)
self.setCentralWidget(main_widget)
def load_image(self):
options = QFileDialog.Options()
file_path, _ = QFileDialog.getOpenFileName(self, "Select an image", "", "All Files (*);;JPG (*.jpg)", options=options)
if file_path:
img_qt = QImage(file_path)
self.image_label.setPixmap(QPixmap.fromImage(img_qt))
self.processed_img = cv2.imread(file_path)
def scale_image(self):
# 实现缩放功能...
pass
def rotate_image(self):
# 实现旋转功能...
pass
def flip_image(self):
# 实现翻转功能...
pass
if __name__ == "__main__":
app = QApplication([])
window = ImageProcessor()
window.show()
app.exec_()
```
在这个例子中,`load_image`方法用于加载图片,而`scale_image`, `rotate_image`, 和 `flip_image`方法应该分别添加具体的图像变换函数,例如使用OpenCV提供的`resize`, `getRotationMatrix2D`, 和 `flip`等函数。然而,为了完整展示这些方法,我会提供一个简化的缩放和旋转示例:
```python
def scale_image(self):
scale_percent = int(self.scale_line_edit.text())
width = int(self.image_label.width() * scale_percent / 100)
height = int(self.image_label.height() * scale_percent / 100)
resized_img = cv2.resize(self.processed_img, (width, height), interpolation=cv2.INTER_LINEAR)
self.update_image(resized_img)
def rotate_image(self):
angle_degrees = int(self.rotate_line_edit.text())
rotation_matrix = cv2.getRotationMatrix2D((self.processed_img.shape[1] // 2, self.processed_img.shape[0] // 2), angle_degrees, 1.0)
rotated_img = cv2.warpAffine(self.processed_img, rotation_matrix, (self.processed_img.shape[1], self.processed_img.shape[0]))
self.update_image(rotated_img)
def update_image(self, new_img):
img_qt = QImage(new_img.data, new_img.shape[1], new_img.shape[0], QImage.Format_RGB888)
pixmap = QPixmap.fromImage(img_qt)
self.image_label.setPixmap(pixmap)
```
注意,这只是一个基本框架,实际应用中还需要添加更多的错误检查和用户体验优化。在每个按钮点击事件后,记得先保存原始图片以便于后续的变换。
阅读全文
相关推荐
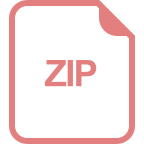
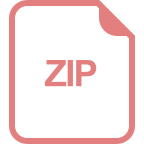
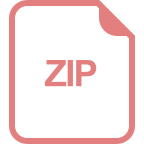
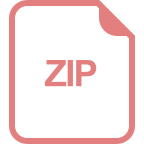
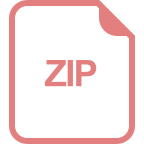
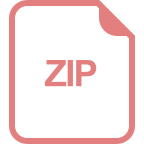
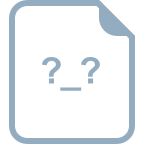
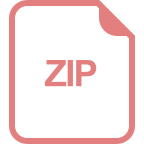
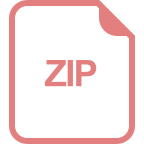
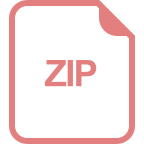
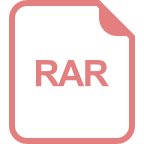
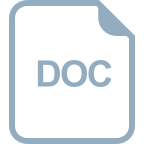



