输入一个字符串,内有数字和非数字字符,如: A123x456 17960?302tab5876,将其中连续的数字作为一个整数,依次用数组存放这些数,统计有多少个整数,并输出这些数。 用子函数实现两个字符数组比大小的功能。用c语言
时间: 2024-12-15 20:16:24 浏览: 31
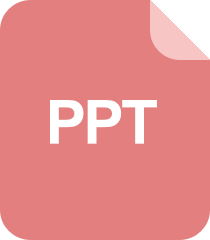
字符型常量-选择文件 ( 03 C++基本要素
要解决这个问题,你可以按照以下步骤编写C语言程序:
1. 首先,我们需要定义一个函数来分割输入的字符串并提取连续的数字:
```c
#include <stdio.h>
#include <ctype.h>
// 函数用于找出字符串中的连续数字序列
int* extractNumbers(char str[], int* count) {
char num[20]; // 存储当前数字
int numIndex = 0;
int arrIndex = 0;
while (str[arrIndex]) {
if (isdigit(str[arrIndex])) { // 如果字符是数字
num[numIndex++] = str[arrIndex];
if (numIndex == 20) { // 数字超过最大长度,清零并开始新一次存储
numIndex = 0;
if (*count != 0)
++(*count); // 数组已满,增加计数
else
*count = 1; // 首个数字
}
} else { // 遇到非数字字符,结束当前数字的收集
num[numIndex] = '\0'; // 添加终止符
*(arrIndex + *count++) = atoi(num); // 转换数字并存入结果数组
numIndex = 0;
}
++arrIndex;
}
// 处理最后一个可能的数字
if (numIndex > 0) {
num[numIndex] = '\0';
*(arrIndex + *count) = atoi(num);
}
return &(*(arrIndex+*count)); // 返回指向结果数组的指针
}
// 比较两个整数数组的函数
int compareArrays(int a[], int b[], int n) {
for (int i = 0; i < n; i++) {
if (a[i] < b[i])
return -1;
else if (a[i] > b[i])
return 1;
}
return 0; // 如果数组相等
}
int main() {
char str[] = "A123x456 17960?302tab5876";
int numCount = 0;
int* numbers = extractNumbers(str, &numCount);
printf("There are %d integers in the string:\n", numCount);
for (int i = 0; i < numCount; i++)
printf("%d ", numbers[i]);
// 示例对比两个数组
int arr1[] = {1, 2, 3};
int arr2[] = {4, 5, 6};
int result = compareArrays(arr1, arr2, 3);
if (result < 0)
printf("\nArray 1 is smaller than Array 2.\n");
else if (result > 0)
printf("\nArray 1 is larger than Array 2.\n");
return 0;
}
```
在这个程序中,我们首先定义了一个函数`extractNumbers`用于提取连续数字,然后定义了`compareArrays`函数来比较两个整数数组。在`main`函数中,我们测试了这两个功能并展示了如何使用它们。注意,这里假设数组大小足够大,实际应用中可能需要动态分配空间。
阅读全文
相关推荐
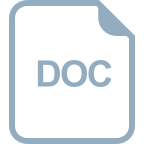
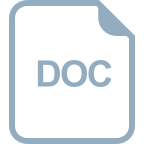














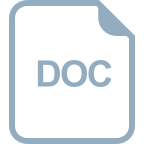