数据结构C语言分别实现顺序表和链表存储学生信息
时间: 2024-09-29 16:05:56 浏览: 11
在 C 语言中,我们可以使用数组来实现顺序表(Sequential List),而链表则更适用于链式存储结构。下面是这两种数据结构分别用于存储学生信息的基本实现:
**1. 顺序表(数组实现)**:
```c
typedef struct {
char name[50]; // 学生姓名
int id; // 学生ID
float score; // 成绩
} Student;
// 顺序表全局变量
Student sequence_list[MAX_STUDENTS];
// 插入或更新学生信息
void add_student(char *name, int id, float score) {
int index = 0;
while (index < MAX_STUDENTS && sequence_list[index].id != '\0') {
index++;
}
if (index < MAX_STUDENTS) {
strcpy(sequence_list[index].name, name);
sequence_list[index].id = id;
sequence_list[index].score = score;
} else {
printf("列表已满,无法添加更多学生。\n");
}
}
// 访问学生信息
void print_student_info(int index) {
printf("Name: %s, ID: %d, Score: %.2f\n", sequence_list[index].name, sequence_list[index].id, sequence_list[index].score);
}
```
**2. 链表(链式实现)**:
```c
typedef struct Node {
char name[50];
int id;
float score;
struct Node* next; // 指向下一个节点的指针
} Node;
// 链表头节点
Node* head = NULL;
// 添加新学生到链表
void add_student_to_linked_list(char* name, int id, float score) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->name = name;
newNode->id = id;
newNode->score = score;
newNode->next = head;
head = newNode;
} else {
printf("内存分配失败。\n");
}
}
// 遍历链表打印学生信息
void print_linked_list() {
Node* current = head;
while (current != NULL) {
printf("Name: %s, ID: %d, Score: %.2f\n", current->name, current->id, current->score);
current = current->next;
}
}
```
相关推荐
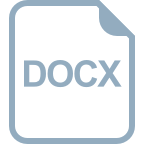
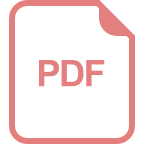
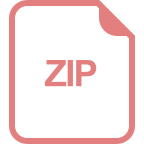
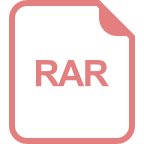
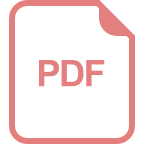
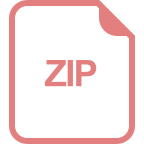
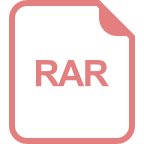
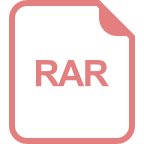
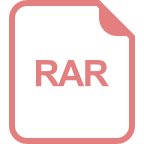
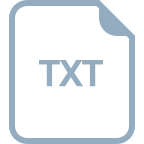
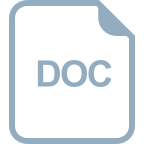
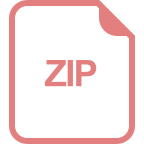
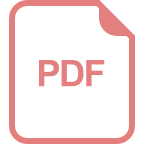
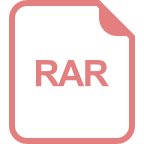
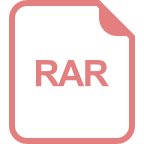
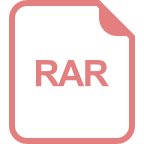
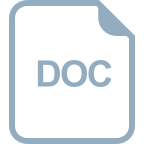
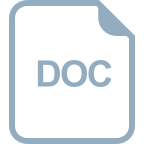
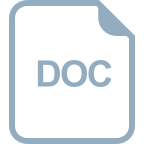