使用C语言编写一个程序来判断二维数组a[1...m,1...n]中的所有元素是否互不相同,并输出相关信息(yes/no)。
时间: 2024-11-04 07:10:21 浏览: 24
为了编写一个C语言程序来判断二维数组中的所有元素是否互不相同,你可以使用一个哈希集合(如`unordered_set`或`std::set`),遍历数组并检查每个元素是否已经存在于集合中。如果找到相同的元素,说明数组中有重复,返回`no`;反之,全部元素唯一,则返回`yes`。
以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdbool.h>
#include <unordered_set>
bool areElementsUnique(int a[][n], int m, int n) {
std::unordered_set<int> uniqueSet;
bool result = true;
for (int i = 0; i < m; ++i) {
for (int j = 0; j < n; ++j) {
if (!uniqueSet.count(a[i][j])) {
uniqueSet.insert(a[i][j]);
} else {
// 如果发现重复元素,更新结果并停止查找
result = false;
break;
}
}
if (!result) {
break;
}
}
return result ? "yes" : "no";
}
int main() {
int a[5][5] = { {1, 2, 3, 4, 5},
{6, 7, 8, 9, 10},
{1, 4, 7, 10, 13},
{1, 4, 7, 10, 13},
{18, 19, 20, 21, 22} };
int m = sizeof(a) / sizeof(a[0]), n = sizeof(a[0]) / sizeof(a[0][0]);
printf("Are all elements in the array unique? %s\n", areElementsUnique(a, m, n));
return 0;
}
```
在这个程序中,`areElementsUnique`函数接受一个二维数组、行数和列数作为输入,然后检查所有元素是否都出现在哈希集合中。如果遇到重复,它将`result`设置为`false`并结束循环。最后,根据`result`的值返回相应的字符串。
阅读全文
相关推荐
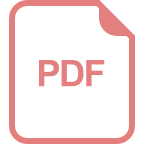
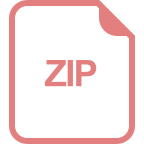
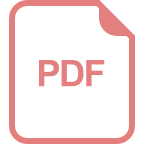
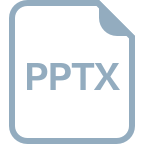
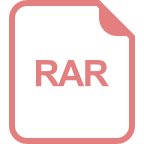
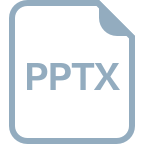
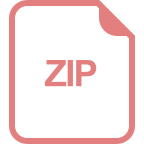
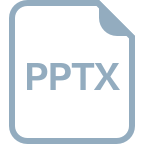
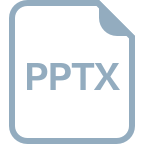
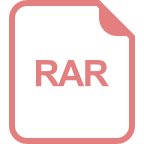
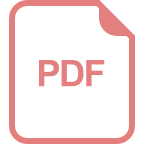
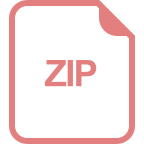
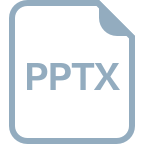
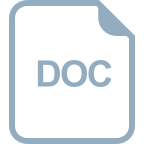
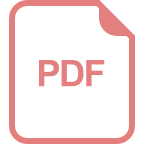
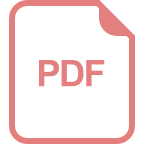
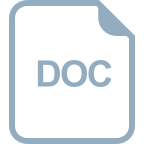