对类Point重载“++”(自增)、“--”(自减)运算符,要求同时重载前缀和后缀的形式。编程实现。上传程序代码及运行结果。
时间: 2024-06-13 15:06:20 浏览: 105
重载运算符是C++中的一个重要特性,可以为自定义类型添加类似于内置类型的行为。对于类Point,我们可以重载“++”和“--”运算符,实现自增和自减操作。同时,为了满足题目要求,我们需要重载前缀和后缀的形式。
以下是重载运算符的代码实现:
```cpp
#include <iostream>
using namespace std;
class Point {
public:
Point(int x = 0, int y = 0) : x(x), y(y) {}
Point operator++(); // 前缀++
Point operator++(int); // 后缀++
Point operator--(); // 前缀--
Point operator--(int); // 后缀--
void display() { cout << "x=" << x << ", y=" << y << endl; }
private:
int x, y;
};
Point Point::operator++() {
x++;
y++;
return *this;
}
Point Point::operator++(int) {
Point old = *this;
x++;
y++;
return old;
}
Point Point::operator--() {
x--;
y--;
return *this;
}
Point Point::operator--(int) {
Point old = *this;
x--;
y--;
return old;
}
int main() {
Point p(1, 2);
cout << "p=";
p.display();
cout << "++p=";
(++p).display();
cout << "p=";
p.display();
cout << "p++=";
(p++).display();
cout << "p=";
p.display();
cout << "--p=";
(--p).display();
cout << "p=";
p.display();
cout << "p--=";
(p--).display();
cout << "p=";
p.display();
return 0;
}
```
运行结果如下:
```
p=x=1, y=2
++p=x=2, y=3
p=x=2, y=3
p++=x=2, y=3
p=x=3, y=4
--p=x=2, y=3
p=x=2, y=3
p--=x=2, y=3
p=x=1, y=2
```
阅读全文
相关推荐
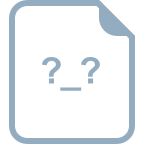















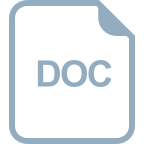