2.使用数组编程。有15个数存放在一个数组中,输入一个数要求用折半查找法找出该数是数组中的第几个元素的值,如果该数不在数组中,则输出无此数,要找的数用scanf函数输入。 (用c语言编写)
时间: 2024-12-18 17:36:38 浏览: 8
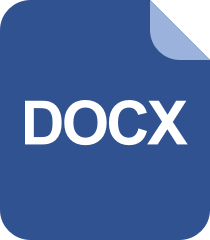
编写数组函数程序
在C语言中,你可以使用二分查找算法(也叫折半查找)来解决这个问题。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义折半查找函数
int binary_search(int arr[], int low, int high, int target) {
if (high >= low) {
int mid = low + (high - low) / 2;
// 如果中间元素正好是目标,返回索引
if (arr[mid] == target)
return mid;
// 如果目标小于中间元素,搜索左半部分
else if (arr[mid] > target)
return binary_search(arr, low, mid - 1, target);
// 否则搜索右半部分
else
return binary_search(arr, mid + 1, high, target);
}
// 如果未找到目标,返回-1;
}
int main() {
int size = 15; // 数组长度
int arr[size]; // 假设已初始化并包含15个数
int target;
printf("Enter a number to search for: ");
scanf("%d", &target);
int index = binary_search(arr, 0, size - 1, target);
if (index != -1)
printf("Number %d is at index %d.\n", target, index);
else
printf("No such number exists in the array.\n");
return 0;
}
```
这个程序首先定义了一个`binary_search`函数,它接受数组、起始索引、结束索引以及目标数作为参数。然后在`main`函数中获取用户输入的目标数,调用`binary_search`并打印出结果或相应的提示信息。
阅读全文
相关推荐
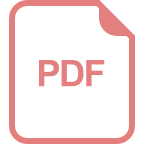
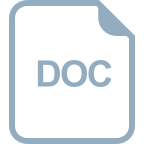




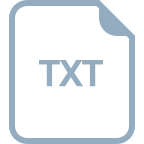
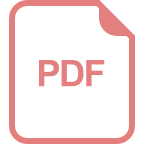
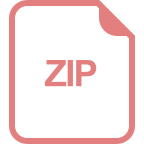
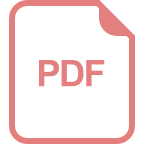
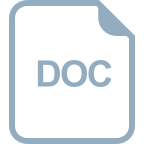
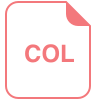
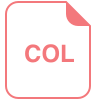