MapReduce综合应用案例 — 招聘数据清洗具体代码
时间: 2024-11-18 20:10:59 浏览: 39
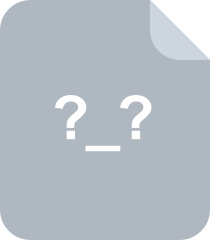
MapReduce综合应用案例 - 招聘数据清洗-结果
MapReduce是一种分布式计算模型,常用于处理大规模数据集。在招聘数据清洗场景中,我们可以利用它来提取、转换和加载大量简历信息。这里提供一个简单的Hadoop MapReduce伪代码示例,假设我们有一个包含应聘者信息的CSV文件,需要清洗如年龄过小、职位不存在等问题:
```java
// Mapper阶段(map函数)
public class ResumeMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text name;
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] fields = value.toString().split(",");
if (isValidAge(fields[AGE_INDEX]) && isValidJob(fields[JOB_INDEX])) {
name.set(fields[NAMES_INDEX]);
context.write(name, one);
}
}
// 辅助方法判断年龄和职位是否有效
private boolean isValidAge(String age) { ... }
private boolean isValidJob(String job) { ... }
}
// Reducer阶段(reduce函数)
public class ResumeReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
private IntWritable count = new IntWritable();
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int total = 0;
for (IntWritable val : values) {
total += val.get();
}
count.set(total);
context.write(key, count);
}
}
// 示例主程序启动MR任务
public static void main(String[] args) throws Exception {
JobConf conf = new JobConf(ResumeJob.class);
conf.setInputFormat TextInputFormat.class;
conf.setOutputKeyClass(Text.class);
conf.setOutputValueClass(IntWritable.class);
conf.setMapperClass(ResumeMapper.class);
conf.setReducerClass(ResumeReducer.class);
FileInputFormat.addInputPath(conf, new Path("input/resumes.csv"));
FileOutputFormat.setOutputPath(conf, new Path("output/cleaned-resumes"));
Job job = Job.getInstance(conf, "Resume Cleaner");
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
```
在这个例子中,Mapper读取输入文件中的每一行简历,检查年龄和职位字段是否有效。如果有效,就将姓名作为键值对输出。Reducer接收这些键(姓名),并对每个姓名的计数求和,得到经过清洗的有效简历总数。
阅读全文
相关推荐
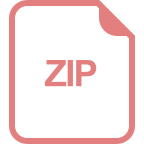
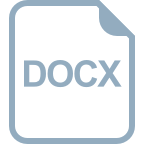

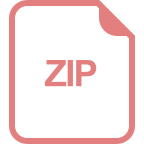
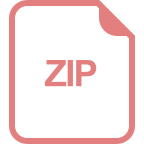
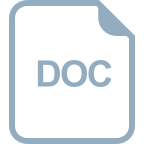
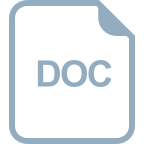
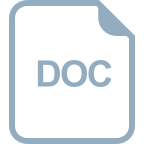
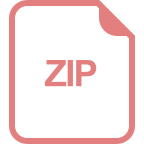
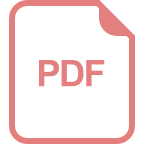
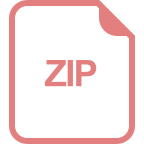
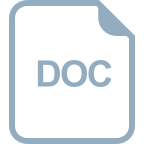
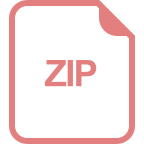
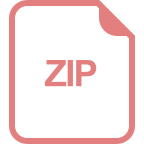
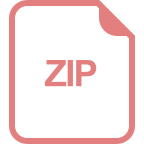
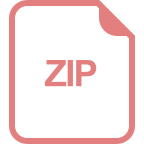