shape = 0.782178018281554 scale = 0.02012987846163316 用这两个参数画出威布尔分布的图像
时间: 2024-04-11 07:33:29 浏览: 94
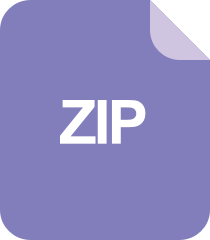
基于Matlab模拟风电场风速两参数weibull(威布尔)分布.zip
当使用shape和scale参数来绘制威布尔分布的图像时,可以使用Python中的SciPy库来完成。下面是使用这两个参数绘制威布尔分布图像的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import weibull_min
shape = 0.782178018281554
scale = 0.02012987846163316
x = np.linspace(0, 10, 100)
y = weibull_min.pdf(x, shape, scale)
plt.plot(x, y)
plt.xlabel('x')
plt.ylabel('Probability Density')
plt.title('Weibull Distribution')
plt.grid(True)
plt.show()
```
以上代码将生成一个威布尔分布的概率密度函数图像,其中x轴表示变量的取值,y轴表示对应取值的概率密度。
请注意,这里的示例代码假设x的取值范围为0到10,你可以根据实际需求调整x的范围。
阅读全文
相关推荐
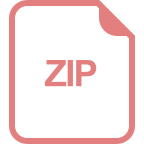


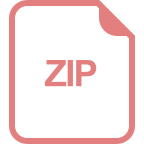
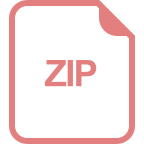
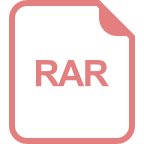
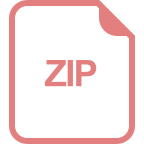
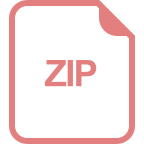

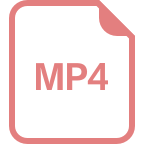
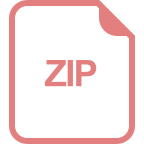
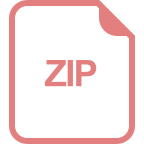