androbench 代码实现
时间: 2024-02-10 07:08:03 浏览: 207
AndroBench是一个Android平台上的存储性能测试工具,它可以测试存储设备的读写速度、随机读写速度、SQLite数据库性能等。下面是一个简单的C语言实现AndroBench的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define FILE_SIZE 1024*1024*10 // 10MB
#define BLOCK_SIZE 4096 // 4KB
void test_write_speed(char *filename) {
FILE *fp;
char *buf;
int i, j;
clock_t start, end;
double duration, speed;
buf = (char *)malloc(BLOCK_SIZE);
memset(buf, 'A', BLOCK_SIZE);
fp = fopen(filename, "wb");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
return;
}
start = clock();
for (i = 0; i < FILE_SIZE / BLOCK_SIZE; i++) {
fwrite(buf, BLOCK_SIZE, 1, fp);
}
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
speed = (double)FILE_SIZE / duration / 1024 / 1024;
printf("Write speed: %.2f MB/s\n", speed);
fclose(fp);
free(buf);
}
void test_read_speed(char *filename) {
FILE *fp;
char *buf;
int i, j;
clock_t start, end;
double duration, speed;
buf = (char *)malloc(BLOCK_SIZE);
fp = fopen(filename, "rb");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
return;
}
start = clock();
for (i = 0; i < FILE_SIZE / BLOCK_SIZE; i++) {
fread(buf, BLOCK_SIZE, 1, fp);
}
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
speed = (double)FILE_SIZE / duration / 1024 / 1024;
printf("Read speed: %.2f MB/s\n", speed);
fclose(fp);
free(buf);
}
int main(int argc, char *argv[]) {
char *filename = "test.bin";
test_write_speed(filename);
test_read_speed(filename);
return 0;
}
```
这个示例代码实现了AndroBench中的写入和读取测试,它会在当前目录下创建一个10MB的二进制文件,并测试写入和读取这个文件的速度。你可以根据需要修改文件大小和块大小来进行测试。
阅读全文
相关推荐
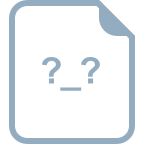
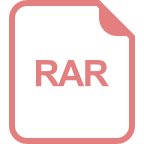
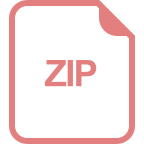
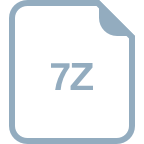
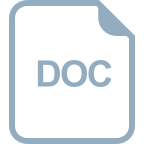
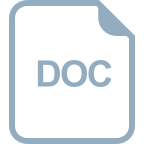


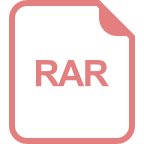
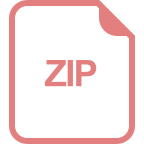
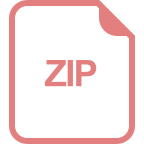
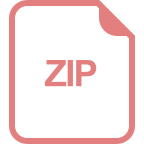
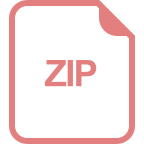
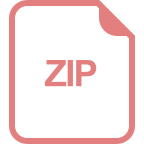
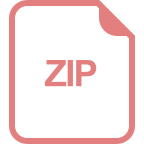