用c语言编写一个程序,利用栈的特点和基本操作,实现如下功能:将一个100以内的正整数N转换为二进制数,并输出转换后的结果。(注:即可采用顺序栈,也可采用链式栈)
时间: 2024-10-22 12:29:26 浏览: 19
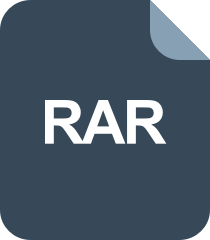
给定一个十进制正整数N,程序输出从1到N的所有整数中,“1”出现的个数。DMU

在C语言中,我们可以使用链式栈(通过结构体定义节点并实现push和pop操作)来实现这个功能。这里是一个简单的链式栈实现的例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点
typedef struct Node {
int value; // 节点值
struct Node* next; // 指向下一个节点的指针
} StackNode;
// 初始化链式栈
StackNode* initStack() {
StackNode* top = (StackNode*)malloc(sizeof(StackNode));
if (top == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
top->value = -1;
top->next = NULL;
return top;
}
// 向栈顶添加元素
void push(StackNode** top, int value) {
StackNode* newNode = (StackNode*)malloc(sizeof(StackNode));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->value = value;
newNode->next = *top;
*top = newNode;
}
// 从栈顶移除元素并返回其值
int pop(StackNode** top) {
if (*top == NULL) {
printf("Stack is empty.\n");
return -1;
}
int value = (*top)->value;
StackNode* temp = *top;
*top = (*top)->next;
free(temp);
return value;
}
// 主函数
int main() {
StackNode* stackTop = initStack();
int num, binary[100] = {0};
printf("Enter a number between 0 and 99: ");
scanf("%d", &num);
while (num > 0) {
push(&stackTop, num % 2);
num /= 2;
}
printf("The binary representation of the number is: ");
while (stackTop != NULL) {
binary[pop(&stackTop)] = 1;
}
for (int i = 0; i < sizeof(binary); i++) {
if (binary[i]) {
printf("1");
} else {
printf("0");
}
}
printf("\n");
return 0;
}
```
这个程序首先创建一个空的链式栈,然后将输入的数字不断除以2并将余数压入栈中。最后,从栈顶取出元素(余数),对应的二进制位设置为1(实际上我们取反得到0和1),直到栈为空。
阅读全文
相关推荐
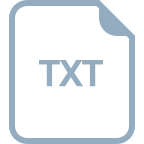
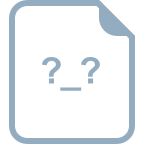



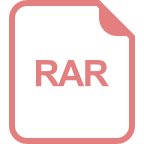
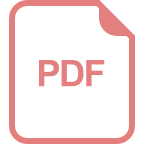
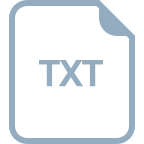
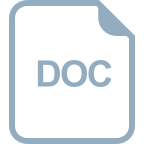
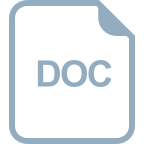
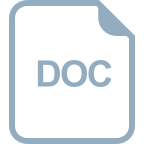
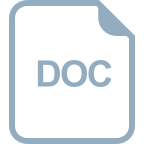
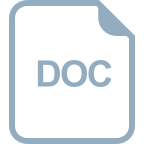
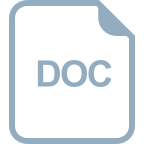
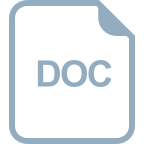
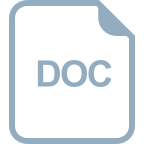