java 读取本地Json文件并返回到浏览器接口的完整代码
时间: 2024-09-06 19:04:41 浏览: 51
在Java中,我们可以使用`java.io.FileReader`和`org.json.JSONObject`库来读取本地JSON文件,并通过Spring Boot创建一个RESTful API将数据返回给浏览器。以下是简单的示例:
首先,假设我们有一个名为`data.json`的本地JSON文件,内容如下:
```json
{
"name": "John",
"age": 30,
"city": "New York"
}
```
然后,在Spring Boot项目中创建一个Controller:
```java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import org.json.JSONObject;
import javax.servlet.http.HttpServletResponse;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
@RestController
public class JsonFileController {
@Value("${file.path:/path/to/data.json}")
private String filePath;
@GetMapping("/api/data")
public ResponseEntity<String> getDataFromJson() throws IOException {
JSONObject jsonObject = readJsonFile(filePath);
return ResponseEntity.ok(jsonObject.toString());
}
private JSONObject readJsonFile(String fileName) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(fileName));
StringBuilder jsonBuilder = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
jsonBuilder.append(line).append('\n');
}
reader.close();
try {
return new JSONObject(jsonBuilder.toString());
} catch (Exception e) {
throw new RuntimeException("Error parsing JSON file", e);
}
}
}
```
在这个例子中,`@GetMapping("/api/data")`表示API的HTTP GET请求,它会调用`getDataFromJson`方法。`readJsonFile`方法用于读取文件并解析为JSONObject。
记得替换`/path/to/data.json`为实际的文件路径,然后运行应用,从浏览器访问`http://localhost:8080/api/data`就能看到转换后的JSON字符串了。
阅读全文
相关推荐
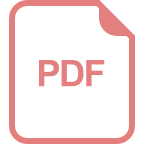
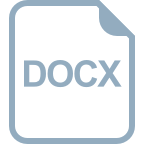
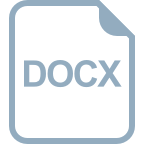



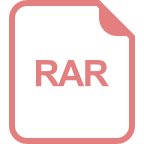
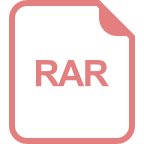
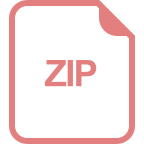
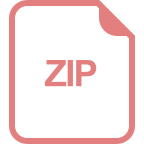
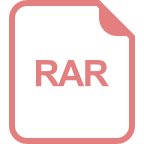
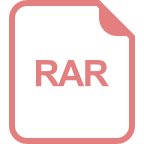
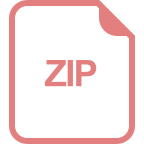
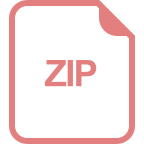
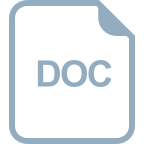
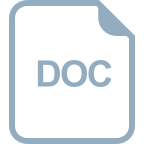


