分析代码功能,并简化
时间: 2024-10-19 21:11:06 浏览: 19
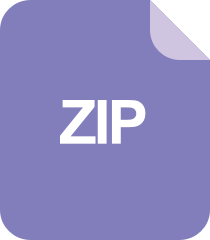
deploy-sonar代码扫描分析工具
### 代码功能分析与简化
#### 主要功能
1. **线性空间和内积空间的定义**:
- `linear_space` 类用于表示线性空间,包含基和数域。
- `inner_product_space` 类继承自 `linear_space`,增加了内积的定义,并实现了施密特正交化方法。
2. **元素的表示**:
- `element` 类用于表示线性空间中的元素,支持坐标表示和值表示之间的转换。
3. **线性变换**:
- `linear_transformation` 类用于表示线性变换,包括线性变换在标准正交基下的矩阵表示和应用线性变换的方法。
4. **坐标变换**:
- `basis_coordinate` 函数用于计算元素在不同基下的坐标。
- `trans_basis_matrix` 函数用于计算线性变换在不同基下的矩阵表示。
5. **具体示例**:
- 计算标准正交基及其上的坐标。
- 计算线性变换在标准正交基和其他基下的矩阵表示。
#### 简化版本
以下是一个简化的版本,保留了主要功能,去除了不必要的细节:
```python
import numpy as np
import copy
class LinearSpace:
def __init__(self, basis=[], number_field=np.complex128):
self.basis = basis
self.number_field = number_field
def dim(self):
return len(self.basis)
class InnerProductSpace(LinearSpace):
def __init__(self, basis=[], number_field=np.complex128, inner_product=None):
super().__init__(basis, number_field)
self.inner_product = inner_product
self.gram_schmidt()
def gram_schmidt(self):
temp_vectors = copy.deepcopy(self.basis)
result = []
for k in range(self.dim()):
current_vector = temp_vectors[k]
norm = np.sqrt(self.inner_product(current_vector, current_vector))
current_vector /= norm
for j in range(k + 1, self.dim()):
projection = self.inner_product(current_vector, temp_vectors[j])
temp_vectors[j] -= projection * current_vector
result.append(current_vector)
self.basis = result
class Element:
def __init__(self, linear_space, info='coordinate', data=[]):
self.linear_space = linear_space
if info == 'coordinate':
self.set_coordinate(data)
elif info == 'value':
self.set_value(data)
def set_coordinate(self, coordinate):
self.coordinate = np.array(coordinate, dtype=self.linear_space.number_field)
def set_value(self, value):
self.coordinate = np.array([self.linear_space.inner_product(value, b) for b in self.linear_space.basis])
def value(self):
v = sum(c * b for c, b in zip(self.coordinate, self.linear_space.basis))
return v
class LinearTransformation:
def __init__(self, linear_space, transformation):
self.linear_space = linear_space
self.transformation = transformation
self.update_matrix()
def update_matrix(self):
transformed_bases = [self.transformation(b) for b in self.linear_space.basis]
self.matrix = np.array([[self.linear_space.inner_product(tb, b) for tb in transformed_bases] for b in self.linear_space.basis])
def apply(self, element):
new_element = Element(self.linear_space, 'coordinate', np.dot(self.matrix, element.coordinate))
return new_element
def change_of_basis(element, new_basis):
old_basis = element.linear_space.basis
C = np.array([Element(element.linear_space, 'value', b).coordinate for b in new_basis]).T
C_inv = np.linalg.inv(C)
new_coordinates = np.dot(C_inv, element.coordinate)
return new_coordinates
def change_of_basis_matrix(transformation, new_basis):
old_matrix = transformation.matrix
C = np.array([Element(transformation.linear_space, 'value', b).coordinate for b in new_basis]).T
C_inv = np.linalg.inv(C)
new_matrix = np.dot(C_inv, np.dot(old_matrix, C))
return new_matrix
# 示例
if __name__ == '__main__':
basis = [np.array([-1, 1]), np.array([-1, 0]), np.array([0, 1])]
number_field = np.float64
inner_product = lambda x, y: np.sum(x * y)
ls = InnerProductSpace(basis, number_field, inner_product)
x = np.array([4, -4])
x_ele = Element(ls, 'value', x)
print('x在标准正交基下的坐标:', x_ele.coordinate)
new_basis = [np.array([-1, 1]), np.array([-1, 0]), np.array([0, 1])]
new_coordinates = change_of_basis(x_ele, new_basis)
print('x在新基下的坐标:', new_coordinates)
transformation = lambda x: x + np.transpose(x)
lt = LinearTransformation(ls, transformation)
print('线性变换在标准正交基下的矩阵:', lt.matrix)
new_matrix = change_of_basis_matrix(lt, new_basis)
print('线性变换在新基下的矩阵:', new_matrix)
```
### 解释
1. **类结构**:
- `LinearSpace` 和 `InnerProductSpace` 定义了线性空间和内积空间的基本属性和方法。
- `Element` 类用于表示线性空间中的元素,支持坐标和值的相互转换。
- `LinearTransformation` 类用于表示线性变换,包括矩阵更新和应用变换的方法。
2. **坐标变换**:
- `change_of_basis` 函数用于计算元素在不同基下的坐标。
- `change_of_basis_matrix` 函数用于计算线性变换在不同基下的矩阵表示。
3. **示例**:
- 创建了一个内积空间并计算了一组标准正交基。
- 计算了某个元素在标准正交基和其他基下的坐标。
- 计算了线性变换在标准正交基和其他基下的矩阵表示。
这个简化版本保留了核心功能,同时减少了冗余代码,使逻辑更加清晰。
阅读全文
相关推荐
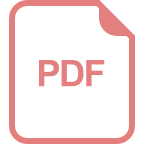
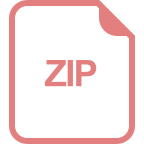
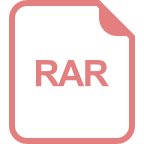
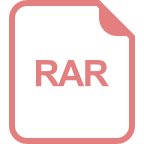
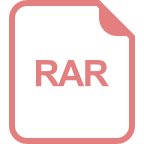
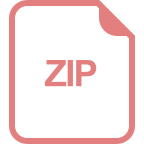
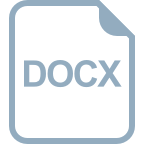
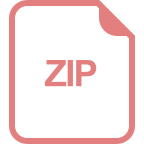
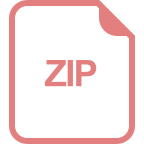
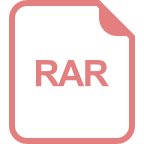
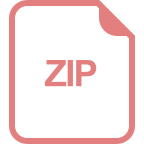
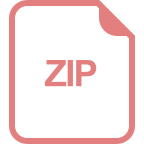
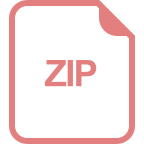
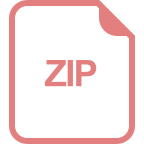
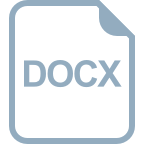
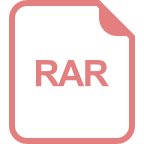
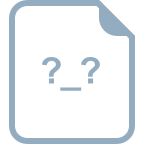
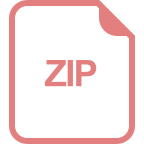