python AES
时间: 2023-11-20 15:57:39 浏览: 91
Python中的AES加密解密需要使用第三方库,常用的有pycryptodome和cryptography等。以下是使用pycryptodome库进行AES加密解密的示例代码:
1. AES加密
```python
from Crypto.Cipher import AES
import base64
# 待加密的明文
text = 'Hello, world!'
# 密钥,必须是16、24或32字节长度
key = b'1234567812345678'
# 使用ECB模式进行加密
cipher = AES.new(key, AES.MODE_ECB)
# 将明文转换成bytes类型,并进行PKCS7填充
text = text.encode('utf-8')
text = text + (16 - len(text) % 16) * chr(16 - len(text) % 16).encode('utf-8')
# 加密
ciphertext = cipher.encrypt(text)
# 将密文进行base64编码
ciphertext = base64.b64encode(ciphertext)
print(ciphertext)
```
2. AES解密
```python
from Crypto.Cipher import AES
import base64
# 待解密的密文
ciphertext = b'Pd04a4bt7Bcf97KEfgLGQw=='
# 密钥,必须是16、24或32字节长度
key = b'1234567812345678'
# 使用ECB模式进行解密
cipher = AES.new(key, AES.MODE_ECB)
# 将密文进行base64解码
ciphertext = base64.b64decode(ciphertext)
# 解密
text = cipher.decrypt(ciphertext)
# 去除PKCS7填充
text = text[:-text[-1]]
# 将明文转换成字符串类型
text = text.decode('utf-8')
print(text)
```
阅读全文
相关推荐
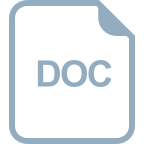
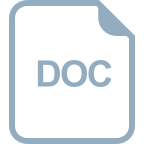
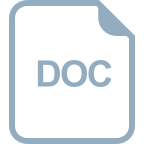














