python去掉指定字符
时间: 2024-11-22 11:35:34 浏览: 4
在Python中,如果你想要去除字符串中的某个特定字符,可以使用`replace()`函数或者利用切片操作。以下是两个简单的方法:
1. 使用 `replace()` 函数:
```python
original_string = "Hello, World!"
char_to_remove = ','
new_string = original_string.replace(char_to_remove, '')
```
在这个例子中,`replace('(',',')`会将所有逗号替换为空字符串,从而删除它们。
2. 切片操作(如果不需要保留原始字符串,可以直接赋值给变量):
```python
original_string = "Hello, World!"
char_to_remove = ','
new_string = original_string[:original_string.index(char_to_remove)] + original_string[original_string.index(char_to_remove) + 1:]
```
这里通过查找指定字符的位置并取两边的部分来实现删除。
相关问题
python去除指定字符
可以使用字符串的replace方法来去除指定字符,例如:
```python
string = "hello world!"
string = string.replace("l", "") # 去除所有的 "l"
print(string) # 输出 "heo word!"
```
另外,还可以使用正则表达式来去除指定字符,例如:
```python
import re
string = "hello world!"
string = re.sub("l", "", string) # 去除所有的 "l"
print(string) # 输出 "heo word!"
```
需要注意的是,这两种方法都是去除所有的指定字符,如果只想去除字符串开头或结尾的指定字符,可以使用字符串的lstrip和rstrip方法。
python 去掉指定字符后的空格
在Python中,如果你想去掉字符串中的指定字符之后的所有空格,你可以使用`replace()`函数先将指定字符替换为空字符串,然后再用`strip()`函数去除多余的空白字符。这里有一个示例:
```python
def remove_spaces_after_char(input_string, char_to_remove):
# 先替换指定字符为无
input_string = input_string.replace(char_to_remove, '')
# 再去除剩余的空格
input_string = input_string.strip()
return input_string
# 示例用法
input_str = "Hello, World! "
char = ','
result = remove_spaces_after_char(input_str, char)
print(result) # 输出:Hello World!
```
在这个例子中,`remove_spaces_after_char`函数接收一个字符串和一个字符作为输入,然后返回处理后的字符串。
阅读全文
相关推荐
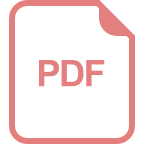
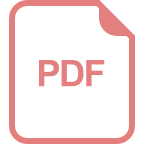
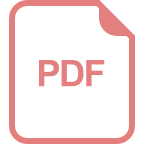




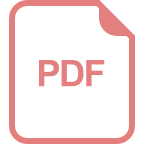








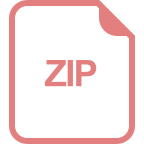