mapreduce实现数据排序
时间: 2023-11-24 17:51:01 浏览: 39
MapReduce是一种分布式计算框架,可以用于对大规模数据进行排序。以下是MapReduce实现数据排序的步骤:
1.编写Map函数和Reduce函数,其中Map函数将输入数据转换为键值对,Reduce函数将键值对按照键进行排序并输出。
2.设置MapReduce作业的输入路径和输出路径。
3.设置MapReduce作业的Mapper类和Reducer类。
4.设置MapReduce作业的输出键值对类型。
5.设置MapReduce作业的排序方式,可以选择部分排序、全排序、辅助排序或二次排序等方式。
6.运行MapReduce作业并等待作业完成。
以下是一个简单的MapReduce排序示例:
```java
// 设置Map函数
public static class Map extends Mapper<LongWritable, Text, IntWritable, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private IntWritable data = new IntWritable();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
data.set(Integer.parseInt(line));
context.write(data, one);
}
}
// 设置Reduce函数
public static class Reduce extends Reducer<IntWritable, IntWritable, IntWritable, IntWritable> {
private IntWritable lineNumber = new IntWritable(1);
public void reduce(IntWritable key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
for (IntWritable val : values) {
context.write(lineNumber, key);
lineNumber = new IntWritable(lineNumber.get() + 1);
}
}
}
// 设置MapReduce作业
Job job = new Job(conf, "sort");
job.setJarByClass(Sort.class);
job.setMapperClass(Map.class);
job.setReducerClass(Reduce.class);
job.setOutputKeyClass(IntWritable.class);
job.setOutputValueClass(IntWritable.class);
job.setInputFormatClass(TextInputFormat.class);
job.setOutputFormatClass(TextOutputFormat.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
// 运行MapReduce作业
job.waitForCompletion(true);
```
相关推荐
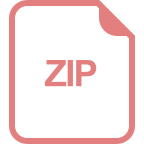
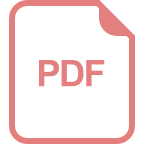
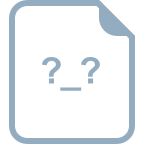














