vue2实现Leaflet在地图上手动绘制多边形,并且获取多边形的经纬度数组
时间: 2024-09-20 13:03:40 浏览: 83
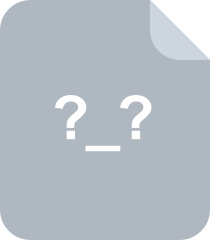
vue2用高德地图实现绘制多边形电子围栏
在 Vue.js 中使用 Leaflet 地图库手动绘制多边形并获取其经纬度数组,你可以按照以下步骤操作:
1. 首先,确保已经在项目中安装了 Vue 和 Leaflet。可以使用 Vue CLI 或手动引入 CDN。
2. 引入依赖:
```html
<script src="https://unpkg.com/leaflet@1.7.1/dist/leaflet.js"></script>
<link rel="stylesheet" href="https://unpkg.com/leaflet@1.7.1/dist/leaflet.css" />
```
3. 在组件里创建一个 Map 组件并初始化 Leaflet 地图:
```js
<template>
<div id="map-container">
<div ref="map" style="height: 500px; width: 100%"></div>
</div>
</template>
<script>
import L from 'leaflet';
export default {
data() {
return {
map: null,
markers: [],
polygon: null,
coordinates: []
};
},
mounted() {
this.initMap();
},
methods: {
initMap() {
this.map = L.map('map-container').setView([51.505, -0.09], 13); // 设置初始中心点和缩放级别
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
attribution: 'Map data © <a href="https://www.openstreetmap.org/">OpenStreetMap</a> contributors',
maxZoom: 18,
}).addTo(this.map);
// 添加点击事件监听器,用于画线
this.map.on('click', (e) => {
const latlng = e.latlng;
if (!this.polygon) {
this.polygon = new L.Polygon([latlng], { color: 'red' });
this.map.addLayer(this.polygon);
this.coordinates.push(latlng);
} else {
this.polygon.setLatLngs([...this.coordinates, latlng]);
this.coordinates.push(latlng);
}
});
},
getPolygonCoordinates() {
return this.coordinates;
}
}
};
</script>
```
在这个例子中,当你点击地图时会添加一个新的顶点到多边形,直到形成一个完整的闭合路径。`getPolygonCoordinates` 方法会在多边形绘制完成后返回所有的经纬度数组。
阅读全文
相关推荐
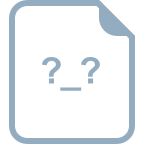
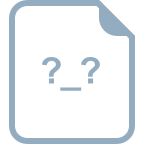
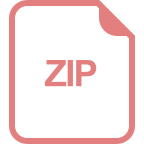
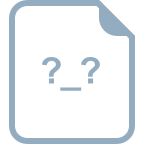
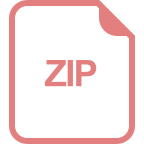
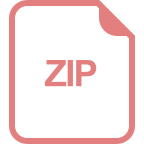
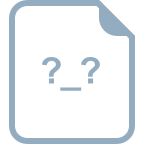
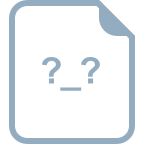
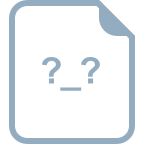
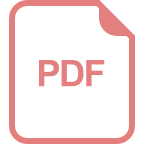
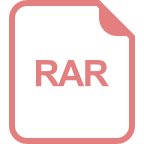
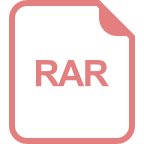
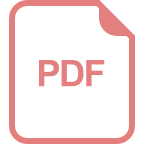
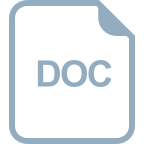
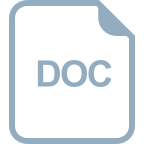
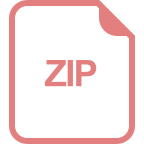