mybatis传入的是一个字符串20240909但是数据库需要的是datetime类型的如何转换
时间: 2024-09-11 21:17:55 浏览: 54
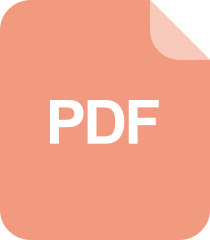
Mybatis3 if判断字符串变态写法
在MyBatis中,如果你需要将传入的字符串转换为数据库中的datetime类型,可以使用MyBatis提供的类型处理器(Type Handlers)。类型处理器用于在Java类型和数据库类型之间进行转换。对于将字符串转换为datetime类型,你可以使用JDBC内置的类型处理器,或者自定义一个类型处理器来实现转换。
以下是一个使用内置类型处理器转换的示例:
1. 使用`#{}`占位符,MyBatis会自动将Java的`Date`对象转换为对应数据库的datetime类型。
```xml
<select id="selectByDate" resultType="YourResultType">
SELECT * FROM your_table WHERE date_column = #{date,jdbcType=TIMESTAMP}
</select>
```
2. 如果你需要手动将字符串转换为`Date`对象,可以先使用Java的`SimpleDateFormat`类来格式化字符串为`Date`对象,然后再使用MyBatis的`#{}`占位符。
```java
String dateString = "20240909";
SimpleDateFormat formatter = new SimpleDateFormat("yyyyMMdd");
Date date = formatter.parse(dateString);
```
然后在MyBatis的映射文件中使用该`Date`对象:
```xml
<select id="selectByDate" resultType="YourResultType">
SELECT * FROM your_table WHERE date_column = #{date}
</select>
```
如果你想确保MyBatis使用特定的转换逻辑,你可以注册一个自定义的类型处理器。这里是一个简单的自定义类型处理器示例:
```java
import java.sql.CallableStatement;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Types;
import org.apache.ibatis.type.JdbcType;
import org.apache.ibatis.type.MappedJdbcTypes;
import org.apache.ibatis.type.MappedTypes;
import org.apache.ibatis.type.TypeHandler;
@MappedTypes(Date.class)
@MappedJdbcTypes({JdbcType.TIMESTAMP, JdbcType.DATE})
public class CustomDateTypeHandler implements TypeHandler<Date> {
@Override
public void setParameter(PreparedStatement ps, int i, Date parameter, JdbcType jdbcType) throws SQLException {
if (parameter == null) {
ps.setNull(i, Types.TIMESTAMP);
} else {
ps.setTimestamp(i, new java.sql.Timestamp(parameter.getTime()));
}
}
@Override
public Date getResult(ResultSet rs, String columnName) throws SQLException {
return rs.getTimestamp(columnName);
}
@Override
public Date getResult(ResultSet rs, int columnIndex) throws SQLException {
return rs.getTimestamp(columnIndex);
}
@Override
public Date getResult(CallableStatement cs, int columnIndex) throws SQLException {
return cs.getTimestamp(columnIndex);
}
}
```
然后在MyBatis配置文件中注册这个类型处理器:
```xml
<typeHandlers>
<typeHandler handler="your.package.CustomDateTypeHandler" />
</typeHandlers>
```
通过上述方法,你可以将传入的字符串转换为数据库中所需的datetime类型。
阅读全文
相关推荐
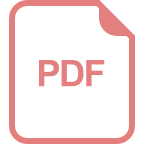
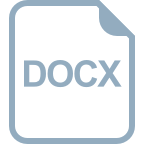
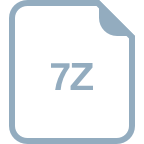
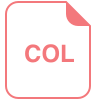
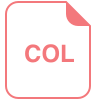








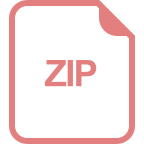
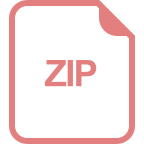