构建一个校园交通查询系统程序, 够规划出任意出发地和目的地之间的最短路径的完整代码。C++语并作出实例说明
时间: 2024-11-22 14:38:11 浏览: 1
构建一个校园交通查询系统的程序涉及到复杂的算法,如图搜索(如Dijkstra's算法、A*算法或Floyd-Warshall算法),以及数据结构(比如邻接矩阵或邻接表)。由于篇幅原因,这里我会给出一个简单的Dijkstra算法的C++示例,用于解决单源最短路径的问题,但请注意这只是一个简化版本,并不适用于大规模复杂网络:
```cpp
#include <iostream>
#include <vector>
#include <queue>
#include <limits>
// 定义边的数据结构
struct Edge {
int src, dest, weight;
};
// 使用优先队列存储节点及其距离
using NodeDistance = std::pair<int, int>;
class Graph {
private:
std::vector<std::vector<Edge>> adjList; // 邻接列表表示
public:
void addEdge(int src, int dest, int weight) {
adjList[src].push_back({src, dest, weight});
}
std::vector<int> dijkstra(int start) {
std::vector<int> distances(adjList.size(), std::numeric_limits<int>::max());
distances[start] = 0;
std::priority_queue<NodeDistance, std::vector<NodeDistance>, std::greater<NodeDistance>> pq;
pq.push({start, 0});
while (!pq.empty()) {
int currNode = pq.top().first;
int distCurrNode = pq.top().second;
pq.pop();
if (distances[currNode] < distCurrNode)
continue; // 如果当前节点距离已经被更新过,则跳过
for (const auto& edge : adjList[currNode]) {
int newDist = distCurrNode + edge.weight;
if (newDist < distances[edge.dest]) {
distances[edge.dest] = newDist;
pq.push({edge.dest, newDist});
}
}
}
return distances;
}
};
int main() {
Graph graph;
// 添加校园地图上的节点和边...
graph.addEdge(0, 1, 5);
graph.addEdge(0, 4, 7);
graph.addEdge(1, 2, 2);
graph.addEdge(1, 3, 4);
graph.addEdge(3, 4, 6);
graph.addEdge(4, 5, 8);
std::vector<int> shortestPath = graph.dijkstra(0); // 从节点0开始计算最短路径
for (int i = 1; i < shortestPath.size(); ++i) {
std::cout << "Shortest distance from node 0 to node " << i << ": " << shortestPath[i] << std::endl;
}
return 0;
}
```
这个例子假设了输入是一个无向加权图,`dijkstra`函数会返回从给定起点到其他所有节点的最短距离。为了适应校园交通查询,你需要将地图数据(例如公交车站、步行道等)转化为这样的图。
阅读全文
相关推荐
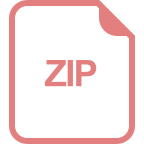
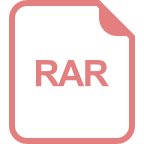
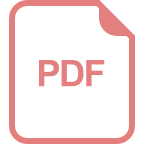
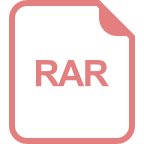
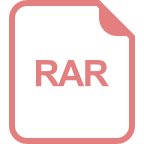
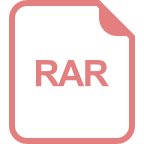
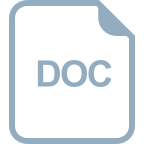
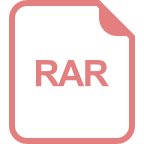
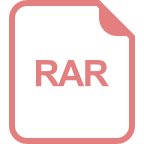
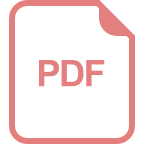
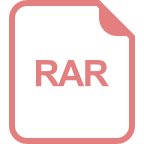