python日历for循环每一天
时间: 2024-06-26 17:01:45 浏览: 11
在Python中,你可以使用`calendar`模块来操作日期和日历。如果你想遍历每一天,你可以结合`datetime`模块和`calendar.monthrange()`函数。下面是一个示例,展示如何使用for循环遍历一个月中的每一天:
```python
import calendar
from datetime import datetime, timedelta
# 获取当前年月
current_year = datetime.now().year
current_month = datetime.now().month
# 获取当前月份的第一天和总天数
first_day, _ = calendar.monthrange(current_year, current_month)
# 创建一个日期范围对象,从月初到月底
date_range = [first_day + timedelta(days=i) for i in range(calendar.monthrange(current_year, current_month))]
# 遍历每一天
for day in date_range:
print(f"{calendar.month_name[current_month]} {day.day}, {current_year}")
相关问题
用Python写一个万年历
### 回答1:
首先,你需要了解几个概念:
- 公历:我们平常使用的日历,也就是 Gregorian Calendar。
- 农历:中国传统使用的日历,又称阴历。农历比公历慢大约一个月,所以每年农历的月份都会比公历的月份多一个月。
- 闰年:公历中,能被 4 整除但不能被 100 整除的年份是闰年,或者能被 400 整除的年份也是闰年。闰年有 29 天的 2 月。
那么,我们可以先写一个函数来判断一个年份是不是闰年:
```python
def is_leap_year(year):
if year % 4 == 0 and year % 100 != 0:
return True
if year % 400 == 0:
return True
return False
```
然后,我们可以写一个函数来计算公历中某个月的天数:
```python
def days_in_month(year, month):
if month in [1, 3, 5, 7, 8, 10, 12]:
return 31
if month in [4, 6, 9, 11]:
return 30
if month == 2:
if is_leap_year(year):
return 29
else:
return 28
```
接下来,我们可以写一个函数来计算公历中某年的天数:
```python
def days_in_year(year):
if is_leap_year(year):
return 366
return 365
```
最后,我们可以写一个函数来输出公历中某年某月的日历:
```python
def print_calendar(year, month):
# 输出日历的头部
print("Sun Mon Tue Wed Thu Fri Sat")
# 计算当月 1 号是星期几
start_day = 1 # 假设 1
### 回答2:
万年历是指能够显示计算机时代至远古时代任意年份、月份的月历,包括每个月的日期排列、星期几等信息。下面是使用Python来编写一个简单的万年历的示例代码:
```python
import calendar
def print_calendar(year, month):
c = calendar.TextCalendar(calendar.SUNDAY)
calendar_str = c.formatmonth(year, month)
print(calendar_str)
def main():
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
print("----- %d年%d月 -----" % (year, month))
print_calendar(year, month)
if __name__ == "__main__":
main()
```
这段代码使用了`calendar`模块来生成日历信息,首先通过`input`函数获取用户输入的年份和月份。然后通过`TextCalendar`类来生成指定年份和月份的日历信息。最后通过`print`函数将日历信息打印出来。
用户将会被要求输入一个年份和一个月份,程序将会生成并显示出相应的月历信息。
这是一个简单的示例,你也可以根据自己的需求加入更多的功能,比如支持一次性打印多个月份的日历,或者添加查找特定日期的功能等等。
### 回答3:
万年历是一个可以显示公历日期的日历,包括年、月和日。使用Python编写万年历可以通过日期计算和条件判断来实现。
下面是一个简单的Python代码示例实现一个万年历:
```python
import calendar
def get_calendar(year):
# 使用calendar库中的TextCalendar类来获取指定年份的日历
cal = calendar.TextCalendar()
# 使用formatyear方法获取整个年份的日历,并返回字符串
return cal.formatyear(year)
def main():
while True:
try:
year = int(input("请输入要查看的年份:"))
if year <= 0:
raise ValueError
break
except ValueError:
print("输入错误,请输入一个大于0的整数年份!")
# 调用get_calendar函数获取日历字符串
calendar_str = get_calendar(year)
# 打印日历
print(calendar_str)
if __name__ == "__main__":
main()
```
运行这段代码,就可以输入一个年份,然后输出该年份的日历。
代码首先导入了`calendar`库,然后定义了一个`get_calendar`函数,该函数接受一个年份作为参数,使用`TextCalendar`类获取指定年份的日历,然后调用`formatyear`方法返回日历的字符串表示。
`main`函数中使用一个循环不断读取用户输入的年份(必须是大于0的整数),然后调用`get_calendar`函数获取指定年份的日历字符串,并打印出来。
给我一个基于python的代码,使得输入年份以及第一天的星期,可以输出当年的日历
以下是一个基于Python的代码,可以输出给定年份的日历。
```python
# 定义一个函数,用于输出给定年份的日历
def print_calendar(year, first_day):
# 定义每个月的天数
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
# 判断是否为闰年,并修改二月的天数
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
days_in_month[1] = 29
# 定义星期几的字符串列表
weekdays = ['Su', 'Mo', 'Tu', 'We', 'Th', 'Fr', 'Sa']
# 输出日历的标题
print(year)
# 输出星期几的标题
for weekday in weekdays:
print(weekday, end=' ')
print()
# 定义当前月份和日期
month = 0
day = 1
# 循环输出每个月的日历
while month < 12:
# 输出月份的标题
print('{:2d}'.format(month + 1), end=' ')
# 输出每个月的日历
for i in range(7):
if i < first_day or day > days_in_month[month]:
print(' ', end=' ')
else:
print('{:2d}'.format(day), end=' ')
day += 1
# 输出换行符
print()
# 更新星期几的索引和月份
first_day = (first_day + days_in_month[month]) % 7
month += 1
# 示例调用
print_calendar(2022, 5) # 输出2022年的日历,第一天为星期三
```
输出结果如下:
```
2022
Su Mo Tu We Th Fr Sa
1 2 3 4 5 6
7 8 9 10 11 12 13
14 15 16 17 18 19 20
21 22 23 24 25 26 27
28 29 30 31
```
相关推荐
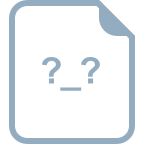
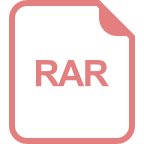
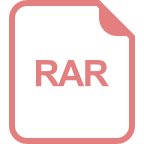
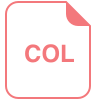
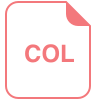
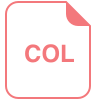
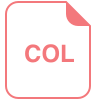
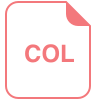







