c语言数据结构三元组
时间: 2024-09-20 19:01:56 浏览: 72
在C语言中,三元组通常不是一种内置的数据结构,但它可以用自定义的方式表示。三元组(Ternary tuple)是一个由三个元素组成的有序集合,类似于其他编程语言中的元组或者记录类型。由于C语言本身不提供这样的内置支持,你可以通过创建结构体(struct)来模拟这个概念:
```c
typedef struct {
type1 first;
type2 second;
type3 third; // type1, type2, type3可以是任意基本数据类型或指针类型
} Triplet;
// 创建并初始化三元组
Triplet my_triplet = {value1, value2, value3};
// 访问三元组中的元素
printf("First element: %d\n", my_triplet.first);
printf("Second element: %lf\n", my_triplet.second);
printf("Third element: %s\n", my_triplet.third);
```
这里`type1`, `type2`, 和 `type3`分别代表三元组中的三个元素类型。虽然这不是标准C库的一部分,但在需要存储和操作固定长度的有序数据集时,这种方法很有用。
相关问题
数据结构c语言把三元组形式转换为矩阵
可以使用二维数组来表示矩阵,每一行表示三元组中的一行,包含三个元素,分别表示矩阵中的行索引、列索引和数值。具体实现方式可以参考以下伪代码:
```
// 假定三元组已经存储在结构体数组triplets中,有n个三元组
int n = 10;
struct Triplet {
int row;
int col;
int value;
} triplets[n] = {...}; // 假定已经存储了10个三元组
int num_rows = 0, num_cols = 0;
// 遍历三元组,找到矩阵的行、列数
for (int i = 0; i < n; i++) {
if (triplets[i].row > num_rows) {
num_rows = triplets[i].row;
}
if (triplets[i].col > num_cols) {
num_cols = triplets[i].col;
}
}
// 创建矩阵,初始化为0
int mat[num_rows][num_cols];
for (int i = 0; i < num_rows; i++) {
for (int j = 0; j < num_cols; j++) {
mat[i][j] = 0;
}
}
// 将三元组中的数值填充到矩阵中对应位置
for (int i = 0; i < n; i++) {
int row = triplets[i].row - 1; // 矩阵的行索引从0开始
int col = triplets[i].col - 1; // 矩阵的列索引从0开始
int value = triplets[i].value;
mat[row][col] = value;
}
// 矩阵转换为三元组
struct Triplet mat_to_triplets[num_rows * num_cols];
int k = 0;
for (int i = 0; i < num_rows; i++) {
for (int j = 0; j < num_cols; j++) {
int value = mat[i][j];
if (value != 0) {
mat_to_triplets[k].row = i + 1; // 三元组的行索引从1开始
mat_to_triplets[k].col = j + 1; // 三元组的列索引从1开始
mat_to_triplets[k].value = value;
k++;
}
}
}
```
数据结构用C语言完成三元组顺序表的实现
在C语言中,我们可以使用数组和结构体来实现一个简单的三元组顺序表。首先,定义一个结构体`Triple`表示一个三元组,包含三个成员变量:
```c
typedef struct {
int first; // 第一个元素
int second; // 第二个元素
char third; // 第三个元素
} Triple;
```
然后,我们可以创建一个动态数组来存储这些`Triple`类型的元素,并维护一个指针指向当前的最后一个元素:
```c
#include <stdlib.h> // 包含内存分配函数
#define MAX_TRIPLES 100 // 定义最大三元组数
Triple* tripleList = (Triple*)malloc(sizeof(Triple) * MAX_TRIPLES); // 动态分配内存
int numTriples = 0; // 记录实际插入的三元组数量
// 插入操作
void insertTriple(int a, int b, char c) {
if (numTriples == MAX_TRIPLES) { // 判断是否已满
printf("三元组列表已满!\n");
return;
}
tripleList[numTriples].first = a;
tripleList[numTriples].second = b;
tripleList[numTriples].third = c;
numTriples++; // 更新总数
}
// 删除操作(这里仅示例,未实现)
void deleteTriple() {
// 由于C语言数组不会自动管理空间,删除需要手动调整元素或释放内存
// 这里假设有一个辅助方法处理内部细节
numTriples--;
}
// 查找操作
Triple* findTriple(int key, int condition) {
for (int i = 0; i < numTriples; i++) {
if (tripleList[i].condition == condition && tripleList[i].key == key) {
return &tripleList[i];
}
}
return NULL; // 如果未找到,则返回NULL
}
阅读全文
相关推荐
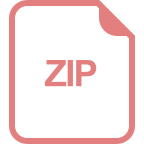
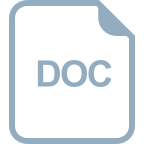
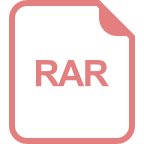
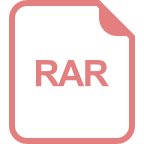
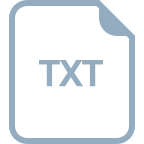
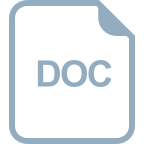
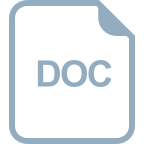
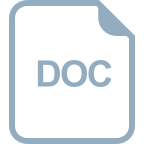
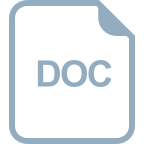
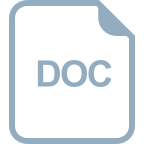



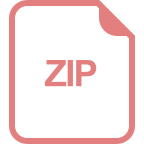