采用分割法算法思想对DFA最小化编程完整实现
时间: 2024-10-24 13:10:40 浏览: 55
分割法是一种用于有限状态自动机(DFA,Deterministic Finite Automaton)最小化的经典算法,它的基本步骤是将DFA分解成多个不可再分的部分,然后合并这些部分,形成一个新的、更小的状态集。以下是使用Python实现的一个简化版的DF阿分裂最小化算法:
```python
from collections import defaultdict
def minimal_DFA_splitting(DFA):
# 初始化数据结构
epsilon_closure = defaultdict(set)
final_states = set()
for state in DFA.states:
if DFA.final[state]:
final_states.add(state)
while True:
new_states = []
changed = False
for state in DFA.states:
if not state in epsilon_closure:
new_state = process_state(DFA, state, epsilon_closure)
new_states.append(new_state)
changed = True
if not changed:
break
# 更新epsilon_closure和final_states
for new_state in new_states:
epsilon_closure.update({new_state: get_epsilon_closure(DFA, new_state)})
DFA.states.extend(new_states)
DFA.transitions = update_transitions(DFA, epsilon_closure)
return DFA.minimize(final_states)
# 辅助函数
def process_state(DFA, state, closure):
new_state = len(DFA.states)
closure[new_state].add(state)
for symbol in DFA.alphabet:
next_state = DFA.transitions[(state, symbol)]
if next_state not in closure:
closure[next_state].add(next_state)
new_state = max(new_state, process_state(DFA, next_state, closure))
return new_state
def get_epsilon_closure(DFA, start_state):
closure = {start_state}
stack = [start_state]
while stack:
current_state = stack.pop()
for next_state in DFA.transitions.get((current_state, ''), []):
if next_state not in closure:
closure.add(next_state)
stack.append(next_state)
return closure
def update_transitions(DFA, epsilon_closure):
new_transitions = {}
for from_state, to_states in DFA.transitions.items():
new_to_states = set()
for to_state in to_states:
if to_state in epsilon_closure[from_state]:
new_to_states.update(epsilon_closure[from_state])
else:
new_to_states.add(to_state)
new_transitions[from_state] = list(new_to_states)
return new_transitions
# 使用示例
# 完整的DFA对象应包含states, alphabet, transitions以及minimize方法
your_dfa = ... # 实际的DFA对象
minimal_dfa = minimal_DFA_splitting(your_dfa)
```
这个实现是一个简化版本,实际应用中可能需要处理更多细节,如错误检查和边界情况。请注意,对于复杂的问题,可能需要引入更专业的库来处理。
阅读全文
相关推荐
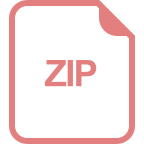
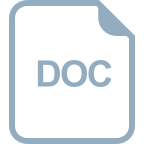

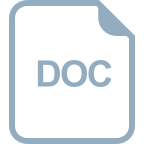
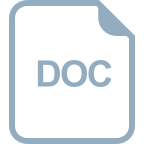
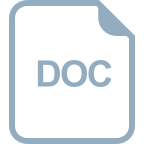
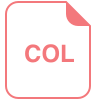
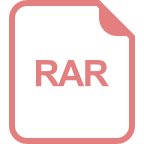
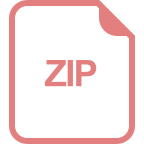
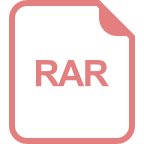
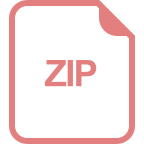
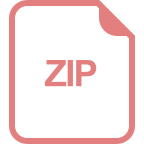
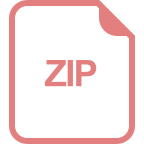
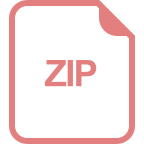