spring-boot-configuration-processor和@value的区别
时间: 2024-11-08 07:01:04 浏览: 7
Spring Boot Configuration Processor是一个工具,它允许你通过注解如`@Value`、`@PropertySource`等直接从配置文件(通常是`.properties`或`.yml`文件)中注入属性到Java Bean中。当你使用`@ConfigurationProperties`注解标注一个类并启用处理器,这个类的字段将自动绑定到相应的配置属性。
`@Value`则是Spring的一个基本特性,用于在运行时动态地设置单个字段的值。例如:
```java
@Configuration
public class AppConfig {
@Value("${my.property}")
private String myProperty;
}
```
在这个例子中,`myProperty`将会读取名为`my.property`的系统环境变量或配置文件中的值。
区别在于:
1. `@ConfigurationProperties`可以处理整个配置对象,而不仅仅是单一字段。
2. `@ConfigurationProperties`支持更复杂的映射规则和默认值、校验等高级功能。
3. `@Value`更为简单,适用于简单的字符串注入,对于复杂的结构化数据,需要手动编写较多的getter和setter。
相关问题
spring-boot-configuration-processor
Spring Boot Configuration Processor is a tool that generates metadata for the configuration properties defined in a Spring Boot application. It helps in providing better documentation and auto-completion features for configuration properties in IDEs like Eclipse and IntelliJ.
When a Spring Boot application is compiled, the Configuration Processor scans the application and generates metadata in the form of a JSON file. This metadata contains information about the configuration properties defined in the application, such as their name, description, default value, and data type.
This metadata can be used by IDEs to provide auto-completion and validation features for configuration properties. For example, if a user starts typing a property name in an IDE, the IDE can show a list of available properties and their descriptions, making it easier for the user to select the correct property.
The Configuration Processor is included in the Spring Boot Starter parent POM, so it is automatically activated when building a Spring Boot application. It can also be explicitly enabled or disabled using configuration properties in the application.properties or application.yml file.
spring-boot-configuration-processor 如何使用
Spring Boot Configuration Processor 是一个用于生成配置元数据的注解处理器,可以帮助我们在编译时生成配置元数据,方便 IDE 自动生成配置属性的提示和文档,提高开发效率。
下面是使用 Spring Boot Configuration Processor 的步骤:
1. 添加 Maven 依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
```
2. 在配置类上添加 `@ConfigurationProperties` 注解,指定配置属性的前缀:
```java
@ConfigurationProperties(prefix = "myconfig")
public class MyConfig {
private String name;
private int age;
// getters and setters
}
```
3. 在配置属性上添加 `@ConfigurationProperties` 注解,指定属性名和默认值:
```java
public class MyConfig {
@ConfigurationProperties("name")
private String name = "default";
@ConfigurationProperties("age")
private int age = 18;
// getters and setters
}
```
4. 在配置类同级目录下创建 `META-INF/spring-configuration-metadata.json` 文件,配置属性的元数据信息:
```json
{
"groups": [
{
"name": "myconfig",
"type": "com.example.demo.MyConfig",
"sourceType": "com.example.demo.MyConfig"
}
],
"properties": [
{
"name": "myconfig.name",
"type": "java.lang.String",
"description": "Name of the user",
"defaultValue": "default"
},
{
"name": "myconfig.age",
"type": "java.lang.Integer",
"description": "Age of the user",
"defaultValue": 18
}
]
}
```
5. 在 IDE 中使用配置属性时,会自动提示和补全属性名、类型、描述和默认值等信息。
注意:在使用 `@ConfigurationProperties` 注解时,需要在配置类上添加 `@EnableConfigurationProperties` 注解,或者在 Spring Boot 应用程序主类上添加 `@EnableConfigurationProperties(MyConfig.class)` 注解,才能使属性生效。
参考文档:https://docs.spring.io/spring-boot/docs/current/reference/html/appendix-configuration-metadata.html#configuration-metadata-annotation-processor
阅读全文
相关推荐
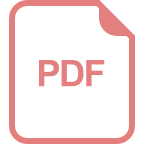
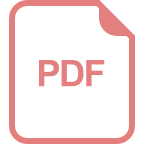
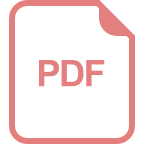



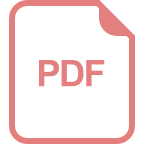
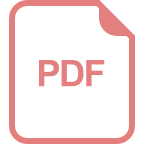
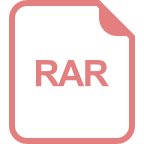
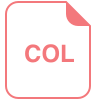
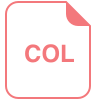
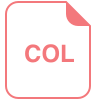
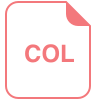
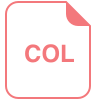
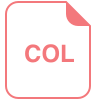


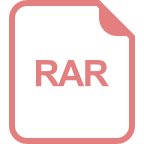