首页命中箭靶Python
命中箭靶Python
时间: 2024-04-19 12:22:38 浏览: 81
命中箭靶是一个基于Python语言的编程游戏,旨在提高编程技能和逻辑思维能力。在游戏中,玩家需要编写代码来控制一个射箭的机器人,使其准确地射中靶子。
游戏的主要特点包括:
1. 编程语言:使用Python语言进行编程,玩家可以通过编写Python代码来控制机器人的行为。
2. 关卡设计:游戏提供了多个关卡,每个关卡都有不同的难度和挑战。玩家需要根据关卡的要求,编写合适的代码来实现射箭的目标。
3. 编辑器界面:游戏提供了一个代码编辑器界面,玩家可以在其中编写和调试代码。编辑器界面通常包括代码输入区域、运行按钮和调试工具等。
4. 反馈机制:游戏会根据玩家编写的代码进行评估和反馈。如果代码正确实现了射箭的目标,游戏会给予相应的奖励和进入下一关的机会;如果代码存在错误或不完整,游戏会给出相应的提示和建议。
5. 学习资源:游戏通常提供了一些学习资源,如教程、示例代码和解题思路等,帮助玩家学习和理解编程的相关知识和技巧。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
大家在看
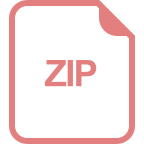
西安石油大学2019-2023 计算机考研808数据结构真题卷
西安石油大学2019-2023 计算机考研808数据结构真题卷,希望能够帮助到大家
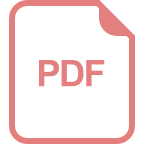
海思芯片规格对比.pdf
本文档介绍了 Hi35XXX 系列芯片,并从芯片的内核、视频编解码性能,图像处理能力,ISP,音频编解码能力,加密引擎,音频接口,外设接口,boot方式,SDK版本,物理特性等进行对比。
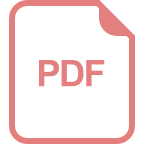
PCIe 6.0官方协议英文版
PCIe协议6.0
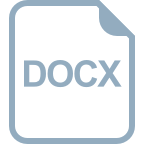
微机原理与嵌入式实验讲义1
注:程序安装完成后,还需要一个器件(pack installer)的安装过程,用来安装相应芯片的开发库和插件等,如图1.1.1所示:图1.1.1 Keil-MD
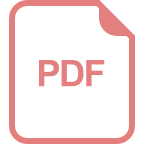
Audio Sink Application Configuration User Guide
CSR的官方文档,主要是介绍用户配置的,以及psr文件的配置项含义。
最新推荐
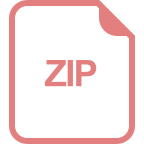
基于java+springboot+mysql+微信小程序的流浪动物救助小程序 源码+数据库+论文(高分毕业设计).zip
项目已获导师指导并通过的高分毕业设计项目,可作为课程设计和期末大作业,下载即用无需修改,项目完整确保可以运行。
包含:项目源码、数据库脚本、软件工具等,该项目可以作为毕设、课程设计使用,前后端代码都在里面。
该系统功能完善、界面美观、操作简单、功能齐全、管理便捷,具有很高的实际应用价值。
项目都经过严格调试,确保可以运行!可以放心下载
技术组成
语言:java
开发环境:idea、微信开发者工具
数据库:MySql5.7以上
部署环境:maven
数据库工具:navicat
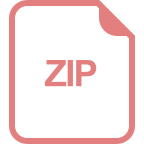
基于springboot的体质测试数据分析及可视化设计源码(java毕业设计完整源码+LW).zip
项目均经过测试,可正常运行!
环境说明:
开发语言:java
JDK版本:jdk1.8
框架:springboot
数据库:mysql 5.7/8
数据库工具:navicat
开发软件:eclipse/idea
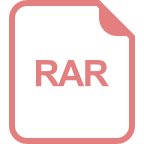
python 3.8.20 windows install 安装包
编译的 python 3.8.20 windows install 安装包
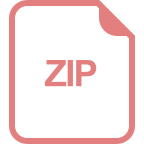
基于go-zero的用户管理系统全部资料+详细文档.zip
【资源说明】
基于go-zero的用户管理系统全部资料+详细文档.zip
【备注】
1、该项目是个人高分项目源码,已获导师指导认可通过,答辩评审分达到95分
2、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用!
3、本项目适合计算机相关专业(人工智能、通信工程、自动化、电子信息、物联网等)的在校学生、老师或者企业员工下载使用,也可作为毕业设计、课程设计、作业、项目初期立项演示等,当然也适合小白学习进阶。
4、如果基础还行,可以在此代码基础上进行修改,以实现其他功能,也可直接用于毕设、课设、作业等。
欢迎下载,沟通交流,互相学习,共同进步!
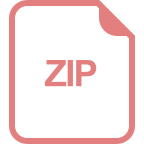
基于springboot的时间管理系统源码(java毕业设计完整源码+LW).zip
时间管理系统采用java技术,基于springboot框架,mysql数据库进行开发,实现了首页,个人中心,系统公告管理,用户管理,时间分类管理,事件数据管理,目标数据管理,用户日记管理等内容进行管理。
环境说明:
开发语言:java
JDK版本:jdk1.8
框架:springboot
数据库:mysql 5.7/8
数据库工具:navicat
开发软件:eclipse/idea
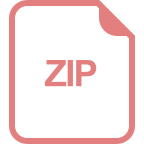
WildFly 8.x中Apache Camel结合REST和Swagger的演示
资源摘要信息:"CamelEE7RestSwagger:Camel on EE 7 with REST and Swagger Demo"
在深入分析这个资源之前,我们需要先了解几个关键的技术组件,它们是Apache Camel、WildFly、Java DSL、REST服务和Swagger。下面是这些知识点的详细解析:
1. Apache Camel框架:
Apache Camel是一个开源的集成框架,它允许开发者采用企业集成模式(Enterprise Integration Patterns,EIP)来实现不同的系统、应用程序和语言之间的无缝集成。Camel基于路由和转换机制,提供了各种组件以支持不同类型的传输和协议,包括HTTP、JMS、TCP/IP等。
2. WildFly应用服务器:
WildFly(以前称为JBoss AS)是一款开源的Java应用服务器,由Red Hat开发。它支持最新的Java EE(企业版Java)规范,是Java企业应用开发中的关键组件之一。WildFly提供了一个全面的Java EE平台,用于部署和管理企业级应用程序。
3. Java DSL(领域特定语言):
Java DSL是一种专门针对特定领域设计的语言,它是用Java编写的小型语言,可以在Camel中用来定义路由规则。DSL可以提供更简单、更直观的语法来表达复杂的集成逻辑,它使开发者能够以一种更接近业务逻辑的方式来编写集成代码。
4. REST服务:
REST(Representational State Transfer)是一种软件架构风格,用于网络上客户端和服务器之间的通信。在RESTful架构中,网络上的每个资源都被唯一标识,并且可以使用标准的HTTP方法(如GET、POST、PUT、DELETE等)进行操作。RESTful服务因其轻量级、易于理解和使用的特性,已经成为Web服务设计的主流风格。
5. Swagger:
Swagger是一个开源的框架,它提供了一种标准的方式来设计、构建、记录和使用RESTful Web服务。Swagger允许开发者描述API的结构,这样就可以自动生成文档、客户端库和服务器存根。通过Swagger,可以清晰地了解API提供的功能和如何使用这些API,从而提高API的可用性和开发效率。
结合以上知识点,CamelEE7RestSwagger这个资源演示了如何在WildFly应用服务器上使用Apache Camel创建RESTful服务,并通过Swagger来记录和展示API信息。整个过程涉及以下几个技术步骤:
- 首先,需要在WildFly上设置和配置Camel环境,确保Camel能够运行并且可以作为路由引擎来使用。
- 其次,通过Java DSL编写Camel路由,定义如何处理来自客户端的HTTP请求,并根据请求的不同执行相应的业务逻辑。
- 接下来,使用Swagger来记录和描述创建的REST API。这包括定义API的路径、支持的操作、请求参数和响应格式等。
- 最后,通过Swagger提供的工具生成API文档和客户端代码,以及服务器端的存根代码,从而使得开发者可以更加便捷地理解和使用这些RESTful服务。
这个资源的实践演示对于想要学习如何在Java EE平台上使用Camel集成框架,并且希望提供和记录REST服务的开发者来说是非常有价值的。通过这种方式,开发者可以更加快速和简单地创建和管理Web服务,同时也增强了API的可访问性和可维护性。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
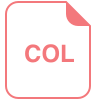
【声子晶体模拟全能指南】:20年经验技术大佬带你从入门到精通
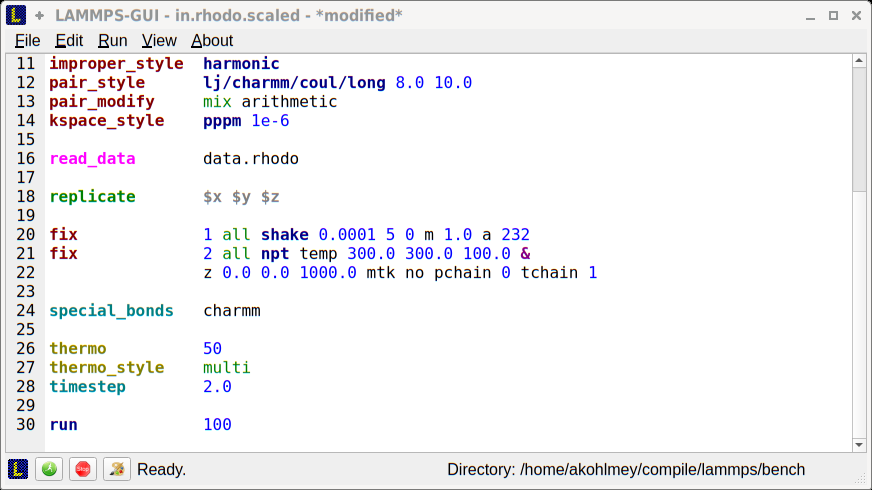
# 摘要
声子晶体作为一种具有周期性结构的材料,在声学隐身、微波和红外领域具有广泛的应用潜力。本文从基础理论出发,深入探讨了声子晶体的概念、物理模型和声子带结构的理论解析,同时介绍了声子晶体的数值模拟方法,包括有限元方法(FEM)、离散元方法(DEM)和分子动力学(MD)。本文还提供了一套完整的声子晶体模拟实践指南,涵盖了模拟前的准备工作、详细的模拟步骤以及结果验证和案例分析。此外,文章探讨了声子晶体模拟的高级技巧和拓展

2024-07-27怎么用python转换成农历日期
在Python中,可以使用`lunarcalendar`库来将公历日期转换为农历日期。首先,你需要安装这个库,可以通过pip命令进行安装:
```bash
pip install lunarcalendar
```
安装完成后,你可以使用以下代码将公历日期转换为农历日期:
```python
from lunarcalendar import Converter, Solar, Lunar, DateNotExist
# 创建一个公历日期对象
solar_date = Solar(2024, 7, 27)
# 将公历日期转换为农历日期
try:
lunar_date = Co
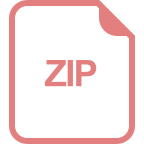
FDFS客户端Python库1.2.6版本发布
资源摘要信息:"FastDFS是一个开源的轻量级分布式文件系统,它对文件进行管理,功能包括文件存储、文件同步、文件访问等,适用于大规模文件存储和高并发访问场景。FastDFS为互联网应用量身定制,充分考虑了冗余备份、负载均衡、线性扩容等机制,保证系统的高可用性和扩展性。
FastDFS 架构包含两个主要的角色:Tracker Server 和 Storage Server。Tracker Server 作用是负载均衡和调度,它接受客户端的请求,为客户端提供文件访问的路径。Storage Server 作用是文件存储,一个 Storage Server 中可以有多个存储路径,文件可以存储在不同的路径上。FastDFS 通过 Tracker Server 和 Storage Server 的配合,可以完成文件上传、下载、删除等操作。
Python 客户端库 fdfs-client-py 是为了解决 FastDFS 文件系统在 Python 环境下的使用。fdfs-client-py 使用了 Thrift 协议,提供了文件上传、下载、删除、查询等接口,使得开发者可以更容易地利用 FastDFS 文件系统进行开发。fdfs-client-py 通常作为 Python 应用程序的一个依赖包进行安装。
针对提供的压缩包文件名 fdfs-client-py-master,这很可能是一个开源项目库的名称。根据文件名和标签“fdfs”,我们可以推测该压缩包包含的是 FastDFS 的 Python 客户端库的源代码文件。这些文件可以用于构建、修改以及扩展 fdfs-client-py 功能以满足特定需求。
由于“标题”和“描述”均与“fdfs-client-py-master1.2.6.zip”有关,没有提供其它具体的信息,因此无法从标题和描述中提取更多的知识点。而压缩包文件名称列表中只有一个文件“fdfs-client-py-master”,这表明我们目前讨论的资源摘要信息是基于对 FastDFS 的 Python 客户端库的一般性了解,而非基于具体文件内容的分析。
根据标签“fdfs”,我们可以深入探讨 FastDFS 相关的概念和技术细节,例如:
- FastDFS 的分布式架构设计
- 文件上传下载机制
- 文件同步机制
- 元数据管理
- Tracker Server 的工作原理
- Storage Server 的工作原理
- 容错和数据恢复机制
- 系统的扩展性和弹性伸缩
在实际使用中,开发者可以通过 fdfs-client-py 库来与 FastDFS 文件系统进行交互,利用其提供的 API 接口实现文件的存储、管理等功能,从而开发出高效、可靠的文件处理应用。开发者可以根据项目的实际需求,选择合适的 FastDFS 版本,并根据官方文档进行安装、配置及优化,确保系统稳定运行。
总的来说,fdfs-client-py 是 FastDFS 文件系统与 Python 应用之间的一座桥梁,它使得开发者能够更加方便地将 FastDFS 集成到基于 Python 开发的应用中,发挥出 FastDFS 在文件管理方面的优势。"