编写一个表单里面包含图书的信息(必须有价格)
时间: 2024-10-13 20:05:30 浏览: 4
当你创建一个包含图书信息的表单时,你可以使用HTML、CSS和JavaScript来构建。这里是一个简单的例子,展示如何创建一个基本的在线图书表单:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>图书信息表单</title>
<style>
/* 表单样式可自定义 */
form {
width: 50%;
max-width: 600px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
}
input, textarea {
width: 100%;
padding: 10px;
margin-bottom: 10px;
box-sizing: border-box;
}
button {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
</style>
</head>
<body>
<form id="bookForm">
<label for="title">书名:</label>
<input type="text" id="title" name="title" required><br>
<label for="author">作者:</label>
<input type="text" id="author" name="author" required><br>
<label for="price">价格:</label>
<input type="number" id="price" name="price" step="0.01" min="0" required><br>
<label for="description">简介:</label>
<textarea id="description" name="description"></textarea><br>
<button type="submit">提交</button>
</form>
<script>
// JavaScript事件处理,例如验证和提交数据
document.getElementById('bookForm').addEventListener('submit', function(e) {
e.preventDefault(); // 阻止表单默认提交行为
const title = document.getElementById('title').value;
const author = document.getElementById('author').value;
const price = document.getElementById('price').value;
// 这里可以添加更多验证或处理逻辑
if (price <= 0) {
alert('请输入有效的价格!');
return;
}
// 提交数据到服务器(实际项目中)
// fetch('https://your-api.com/books', {
// method: 'POST',
// body: JSON.stringify({
// title,
// author,
// price,
// description: document.getElementById('description').value
// }),
// headers: {
// 'Content-Type': 'application/json'
// }
// });
});
</script>
</body>
</html>
```
在这个示例中,我们创建了一个包含书名、作者、价格和描述字段的表单。表单在用户点击“提交”按钮时进行简单验证(确保价格为正数),然后可以选择性地发送数据到服务器。
阅读全文
相关推荐
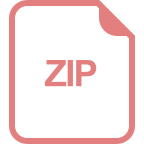
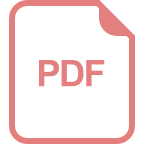
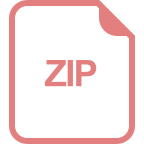















