qt添加自定义XXXPoint类(XXXPoint.h和XXXPoint.cpp都要有各自的内容),并使用该类,使得每点击一次按钮,实现对象参数设置(每按一次,x和y参数自动加1)并打印显示(或在对话框显示)。
时间: 2024-09-28 17:14:54 浏览: 35
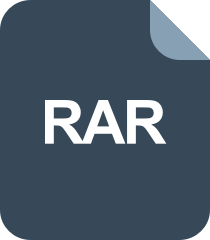
ccrashstack类
为了在Qt应用程序中添加一个名为`CustomPoint`的自定义类,并实现在每次点击按钮时更新和显示它的坐标值,你需要做以下几个步骤:
1. 创建头文件(XXXPoint.h):
```cpp
// CustomPoint.h
#ifndef CUSTOMPOINT_H
#define CUSTOMPOINT_H
#include <QObject>
class CustomPoint : public QObject
{
Q_OBJECT
public:
explicit CustomPoint(QObject *parent = nullptr);
int getX() const;
void setX(int x);
int getY() const;
void setY(int y);
signals:
void positionChanged();
private:
int m_x;
int m_y;
};
#endif // CUSTOMPOINT_H
```
在这个头文件中,我们定义了一个`CustomPoint`类,它是一个`QObject`派生类,包含两个整数成员变量`m_x`和`m_y`表示点的坐标。还定义了getter和setter方法,以及一个信号`positionChanged()`用于通知位置改变。
2. 创建源文件(XXXPoint.cpp):
```cpp
// XXXPoint.cpp
#include "XXXPoint.h"
CustomPoint::CustomPoint(QObject *parent)
: QObject(parent)
{
}
int CustomPoint::getX() const
{
return m_x;
}
void CustomPoint::setX(int x)
{
if (x != m_x) {
m_x = x;
emit positionChanged();
}
}
int CustomPoint::getY() const
{
return m_y;
}
void CustomPoint::setY(int y)
{
if (y != m_y) {
m_y = y;
emit positionChanged();
}
}
```
这里实现了类的构造函数,并且当`x`或`y`属性变化时,会发送`positionChanged`信号。
3. 在主窗口或需要处理这个类的UI组件中使用`CustomPoint`:
```cpp
// 主窗口或其他相关类中
CustomPoint *customPoint = new CustomPoint(this); // 将对象绑定到窗口
QPushButton *button = new QPushButton("Increment", this);
connect(button, &QPushButton::clicked, customPoint, &CustomPoint::setX); // 点击按钮增加x坐标
connect(button, &QPushButton::clicked, customPoint, &CustomPoint::setY); // ...同样连接y坐标
void displayPosition()
{
qDebug() << "Current position: (" << customPoint->getX() << ", " << customPoint->getY() << ")";
// 或者在这里创建一个对话框显示坐标
}
// 每次位置改变时,调用displayPosition函数
QMetaObject::Connection connection = connect(customPoint, &CustomPoint::positionChanged, this, &YourClass::displayPosition);
```
当你按下按钮时,`setX`和`setY`会被调用,坐标值会增加1,并触发`positionChanged`信号,然后`displayPosition`函数会显示新的坐标。
阅读全文
相关推荐
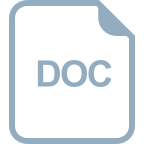
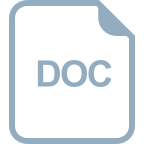



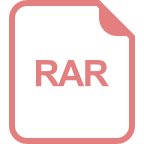
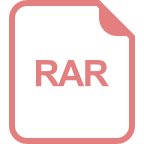
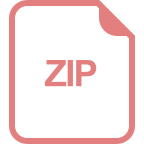
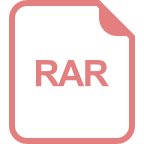
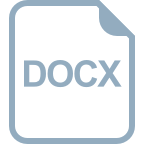
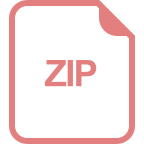
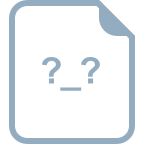
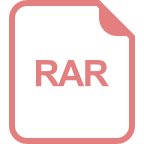
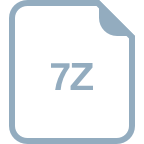
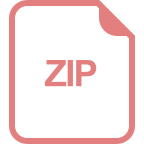