android bitmap上传到服务器
时间: 2024-02-03 07:00:38 浏览: 156
在Android上将Bitmap上传到服务器可以通过以下步骤完成:
1. 将Bitmap转换为字节数组:使用Bitmap的compress()方法将Bitmap对象压缩为JPEG或PNG格式的字节数组。可以根据需要设置压缩质量和格式。
2. 创建HTTP连接并设置请求类型:使用HttpURLConnection类或类似的网络请求类创建连接对象并设置请求类型为POST。
3. 设置连接属性:设置请求的URL、超时时间、缓存模式等连接属性。
4. 设置请求参数:将转换后的字节数组作为请求参数发送给服务器。可以使用URLConnection的getOutputStream()方法将数据写入请求的输出流中。
5. 处理服务器响应:使用URLConnection的getInputStream()方法获取服务器返回的输入流,并读取响应数据。
6. 关闭连接:关闭连接和输入输出流。
以下是一个示例代码:
```java
Bitmap bitmap = BitmapFactory.decodeFile("path_to_image.jpg");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.JPEG, 80, baos);
byte[] imageBytes = baos.toByteArray();
String url = "http://example.com/upload.php";
URL uploadUrl = new URL(url);
HttpURLConnection connection = (HttpURLConnection) uploadUrl.openConnection();
connection.setDoOutput(true);
connection.setRequestMethod("POST");
OutputStream outputStream = connection.getOutputStream();
outputStream.write(imageBytes);
outputStream.flush();
outputStream.close();
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
// 服务器响应成功,进行响应处理
InputStream inputStream = connection.getInputStream();
// 处理服务器返回的数据
inputStream.close();
} else {
// 处理服务器响应失败的情况
}
connection.disconnect();
```
上述代码将Bitmap对象转换为字节数组,然后使用POST方法将数据发送到指定的URL。在实际开发中,你需要替换为自己的服务器URL,并根据实际需求进行适当的修改。
阅读全文
相关推荐
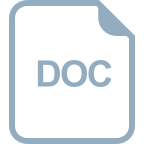
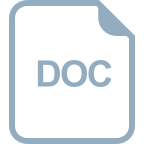
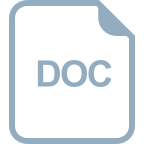

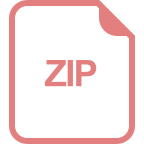
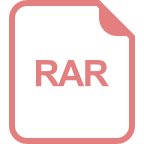
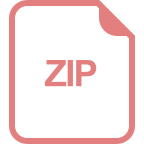
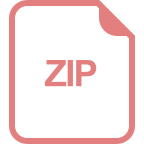
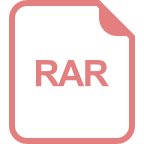
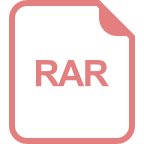
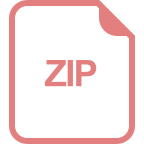
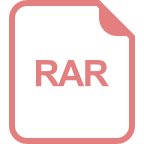
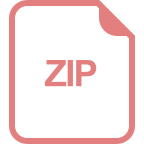
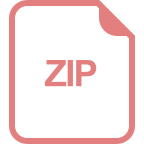
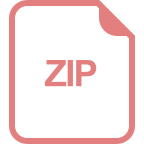
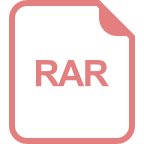
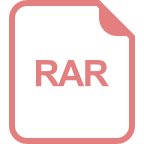
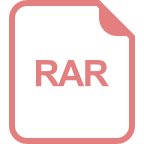
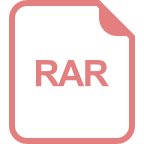