提供一个jdk1.8加密和解密工具类:三重 DES 的加密方法 分组链接方式采用CBC方式,初始向量为8个0x00。 填充方式采用PKCS5-Padding
时间: 2024-12-16 13:21:04 浏览: 2
在Java 8中,我们可以创建一个简单的工具类来实现DES(Data Encryption Standard)的三重加密,采用CBC(Cipher Block Chaining)模式,并使用PKCS5Padding填充。这里是一个基础的示例,首先需要添加Bouncy Castle库的支持,因为它包含DESede(TripleDES)算法:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
public class CryptoUtil {
static {
try {
Security.addProvider(new BouncyCastleProvider());
} catch (Exception e) {
throw new RuntimeException("Error initializing Bouncy Castle provider", e);
}
}
private static final String KEY = "your_secret_key_3des"; // 3DES key,长度为24字节
private static final byte[] IV = {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}; // 初始向量
public static String encrypt(String plainText) throws Exception {
Cipher cipher = Cipher.getInstance("DESede/CBC/PKCS5Padding");
SecretKeySpec secretKey = new SecretKeySpec(KEY.getBytes(), "DESede");
IvParameterSpec iv = new IvParameterSpec(IV);
cipher.init(Cipher.ENCRYPT_MODE, secretKey, iv);
byte[] encryptedBytes = cipher.doFinal(plainText.getBytes("UTF-8"));
return bytesToHex(encryptedBytes);
}
public static String decrypt(String encryptedText) throws Exception {
Cipher cipher = Cipher.getInstance("DESede/CBC/PKCS5Padding");
SecretKeySpec secretKey = new SecretKeySpec(KEY.getBytes(), "DESede");
IvParameterSpec iv = new IvParameterSpec(IV);
cipher.init(Cipher.DECRYPT_MODE, secretKey, iv);
byte[] decryptedBytes = cipher.doFinal(hexToBytes(encryptedText));
return new String(decryptedBytes, "UTF-8");
}
// 字节转十六进制字符串
private static String bytesToHex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02X", b));
}
return sb.toString();
}
// 十六进制字符串转字节数组
private static byte[] hexToBytes(String hexStr) {
int len = hexStr.length();
byte[] data = new byte[len / 2];
for (int i = 0; i < len; i += 2) {
data[i / 2] = (byte) ((Character.digit(hexStr.charAt(i), 16) << 4)
+ Character.digit(hexStr.charAt(i+1), 16));
}
return data;
}
}
// 使用示例
try {
String plaintext = "Your secret message";
String ciphertext = CryptoUtil.encrypt(plaintext);
System.out.println("Encrypted: " + ciphertext);
String decryptedText = CryptoUtil.decrypt(ciphertext);
System.out.println("Decrypted: " + decryptedText);
} catch (Exception e) {
e.printStackTrace();
}
```
在这个例子中,`encrypt`方法用于加密文本,`decrypt`方法用于解密。注意在实际生产环境中,应该妥善管理密钥和初始化向量(IV),避免明文存储或泄露。
阅读全文
相关推荐
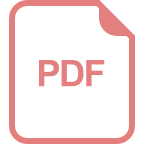
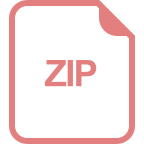
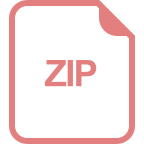
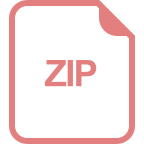
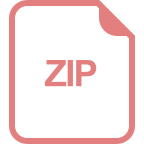
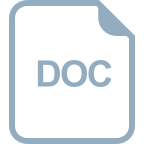
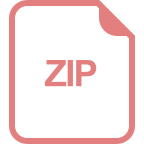
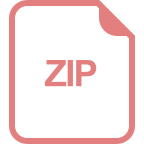
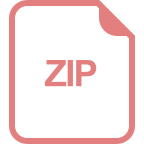
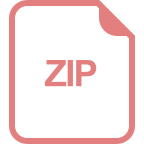
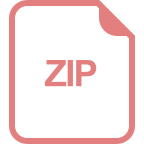
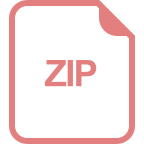
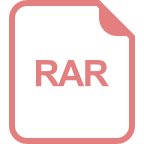
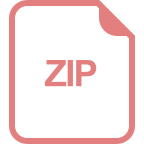
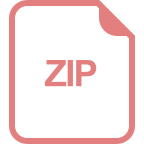
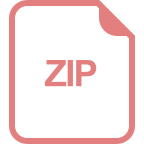
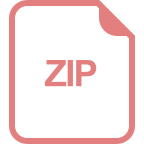