opencv双目圆点标定代码
时间: 2024-01-24 08:15:29 浏览: 145
根据提供的引用内容,我无法找到关于opencv双目圆点标定的具体代码。但是,我可以为您提供一般的步骤和示例代码,以帮助您进行双目相机的标定。
双目相机的圆点标定通常包括以下步骤:
1. 收集标定图像:使用双目相机拍摄一系列标定图像,这些图像应该包含已知的三维点和对应的二维图像点。
2. 提取角点:对于每个标定图像,使用角点检测算法(如OpenCV中的`findChessboardCorners`函数)来检测图像中的角点。
3. 三维-二维对应关系:将每个检测到的角点与其对应的已知三维点进行匹配。
4. 标定相机:使用标定图像和对应的三维-二维对应关系来计算相机的内部参数(如相机矩阵、畸变系数等)和外部参数(如旋转矩阵、平移向量等)。
5. 评估标定结果:使用标定结果对其他图像进行校正和测量。
下面是一个简单的示例代码,演示了如何使用OpenCV进行双目相机的圆点标定:
```python
import cv2
import numpy as np
# 读取标定图像
left_images = [...] # 左相机图像路径列表
right_images = [...] # 右相机图像路径列表
# 设置标定板参数
pattern_size = (9, 6) # 内角点个数
square_size = 1.0 # 棋盘格方块大小(单位:毫米)
# 准备角点容器
obj_points = [] # 三维点
left_img_points = [] # 左相机角点
right_img_points = [] # 右相机角点
# 收集标定图像的角点
for left_img_path, right_img_path in zip(left_images, right_images):
# 读取图像
left_img = cv2.imread(left_img_path)
right_img = cv2.imread(right_img_path)
# 转换为灰度图像
left_gray = cv2.cvtColor(left_img, cv2.COLOR_BGR2GRAY)
right_gray = cv2.cvtColor(right_img, cv2.COLOR_BGR2GRAY)
# 查找角点
left_found, left_corners = cv2.findChessboardCorners(left_gray, pattern_size)
right_found, right_corners = cv2.findChessboardCorners(right_gray, pattern_size)
# 如果两个相机都找到了角点
if left_found and right_found:
# 添加三维点
obj_points.append(np.zeros((pattern_size[0] * pattern_size[1], 3), np.float32))
obj_points[-1][:, :2] = np.mgrid[0:pattern_size[0], 0:pattern_size[1]].T.reshape(-1, 2) * square_size
# 添加左相机角点和右相机角点
left_img_points.append(left_corners)
right_img_points.append(right_corners)
# 进行双目相机标定
ret, left_camera_matrix, left_dist_coeffs, right_camera_matrix, right_dist_coeffs, R, T, E, F = cv2.stereoCalibrate(
obj_points, left_img_points, right_img_points, None, None, None, None, left_gray.shape[::-1], flags=cv2.CALIB_FIX_INTRINSIC)
# 打印标定结果
print("左相机内参矩阵:")
print(left_camera_matrix)
print("左相机畸变系数:")
print(left_dist_coeffs)
print("右相机内参矩阵:")
print(right_camera_matrix)
print("右相机畸变系数:")
print(right_dist_coeffs)
print("旋转矩阵:")
print(R)
print("平移向量:")
print(T)
print("本质矩阵:")
print(E)
print("基础矩阵:")
print(F)
```
请注意,上述代码仅提供了一个基本的示例,实际应用中可能需要根据您的具体需求进行修改和调整。
阅读全文
相关推荐
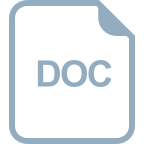
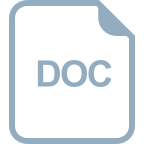
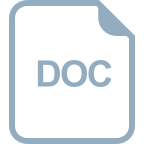
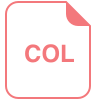
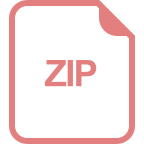
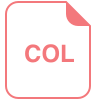
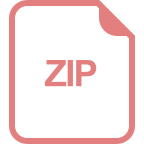
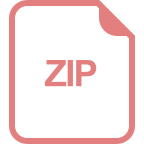
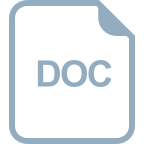
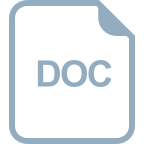
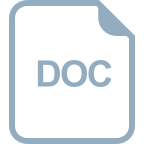
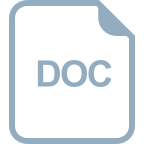
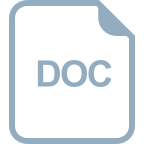
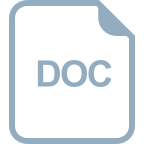
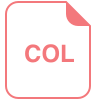
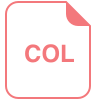
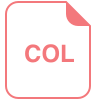
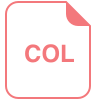