1、根据给定的整型数组,以尾插法建立一个单链表,并实现以下操作: ①查找:输入一个欲查找的整数,找到则显示第一个相匹配的整数在单链表中所处的位置,若不存在,则显示提示信息。
时间: 2024-10-15 07:29:50 浏览: 19
尾插法是指在创建链表时,每次都将新节点添加到链表的末尾。以下是使用Python实现一个简单的单链表结构以及实现查找操作的过程:
首先,我们需要定义链表节点的基本结构:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
```
然后我们创建链表类,其中包含插入和查找方法:
```python
class LinkedList:
def __init__(self):
self.head = None
def append(self, value):
if not self.head:
self.head = Node(value)
else:
current = self.head
while current.next:
current = current.next
current.next = Node(value)
def search(self, target):
current = self.head
position = 0
while current:
if current.data == target:
return f"目标值 {target} 的位置是第 {position + 1} 个"
current = current.next
position += 1
return "目标值不在链表中"
# 示例
linked_list = LinkedList()
numbers = [1, 3, 5, 7, 9]
for num in numbers:
linked_list.append(num)
# 查找示例
print(linked_list.search(5)) # 输出:目标值 5 的位置是第 3 个
print(linked_list.search(10)) # 输出:目标值 10 不在链表中
```
阅读全文
相关推荐
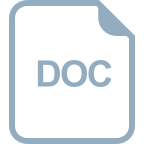
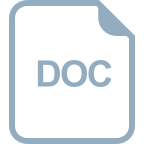
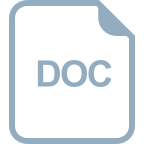
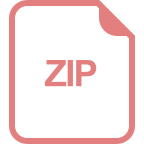
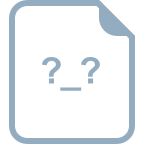
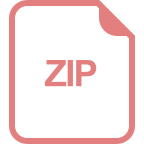
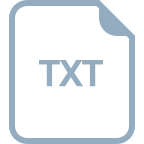
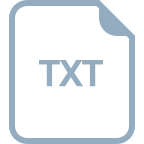
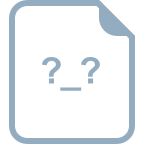
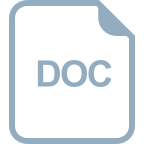
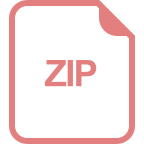
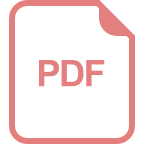
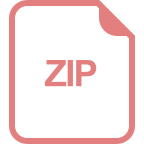
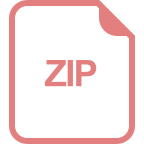
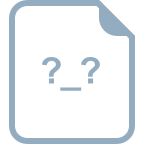
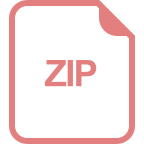
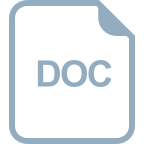
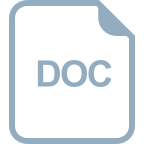
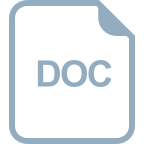