轻量化openpose
时间: 2023-08-31 07:13:23 浏览: 155
轻量化OpenPose是指针对OpenPose算法进行优化,以减少其模型的大小和计算量,从而使其能够在资源受限的设备上运行。下面是一些常见的轻量化OpenPose的方法:
1. MobileNet作为主干网络:将传统的ResNet等较重的主干网络替换为MobileNet,其参数量较小,计算量较低,适合移动端或嵌入式设备。通过调整网络结构和深度可实现更好的平衡。
2. 参数剪枝和量化:使用参数剪枝和量化技术可以减少模型的参数量和内存占用。剪枝可以通过删除不重要的权重或通道来减少参数量,而量化可以将浮点数参数转换为低精度的定点数或整数,从而减少存储和计算开销。
3. 网络结构优化:通过改进网络结构,如减少通道数、减小卷积核大小、减少网络层数等方式,可以降低计算量和模型大小。
4. 单阶段检测:传统的OpenPose算法是两阶段的,包括人体关键点检测和连接生成。而单阶段检测将两个阶段合并为一个网络,可以减少计算量。
5. 实时姿态估计:对于实时应用场景,可以通过限制关键点的搜索范围、降低检测的精度、减少推理帧率等方式来减少计算量,以实现实时性。
这些方法可以根据具体的需求和设备资源进行选择和组合,以实现轻量化OpenPose。
相关问题
如何在lightweight OpenPose人体姿态估计网络中添加SENet注意力模块,代码实现
### 回答1:
在lightweight OpenPose人体姿态估计网络中添加SENet注意力模块,可以参考以下步骤:
1. 首先需要在代码中导入相应的库,如下所示:
```
import torch
import torch.nn as nn
import torch.nn.functional as F
```
2. 定义SENet注意力模块,代码如下:
```
class SEModule(nn.Module):
def __init__(self, channels, reduction):
super(SEModule, self).__init__()
self.avg_pool = nn.AdaptiveAvgPool2d(1)
self.fc1 = nn.Conv2d(channels, channels // reduction, kernel_size=1, bias=False)
self.relu = nn.ReLU(inplace=True)
self.fc2 = nn.Conv2d(channels // reduction, channels, kernel_size=1, bias=False)
self.sigmoid = nn.Sigmoid()
def forward(self, x):
module_input = x
x = self.avg_pool(x)
x = self.fc1(x)
x = self.relu(x)
x = self.fc2(x)
x = self.sigmoid(x)
return module_input * x
```
其中,`channels`表示输入的通道数,`reduction`表示缩减比例。
3. 在lightweight OpenPose人体姿态估计网络中添加SENet注意力模块,代码如下:
```
class PoseEstimationWithSENet(nn.Module):
def __init__(self, num keypoints):
super(PoseEstimationWithSENet, self).__init__()
# 定义网络结构
# ...
# 添加SENet注意力模块
self.se_block1 = SEModule(64, 16)
self.se_block2 = SEModule(128, 16)
self.se_block3 = SEModule(256, 16)
self.se_block4 = SEModule(512, 16)
# 定义输出层
# ...
def forward(self, x):
# 前向传播过程
# ...
# 添加SENet注意力模块
x1 = self.se_block1(x1)
x2 = self.se_block2(x2)
x3 = self.se_block3(x3)
x4 = self.se_block4(x4)
# 输出结果
# ...
return out
```
其中,`num_keypoints`表示需要预测的关键点数量。
在代码中添加SENet注意力模块后,就可以对人体姿态进行更加准确的估计。
### 回答2:
在lightweight OpenPose人体姿态估计网络中添加SENet注意力模块可以通过以下步骤完成:
1. 导入必要的库和模块,包括torch、torchvision、torch.nn等。
2. 定义SEBlock模块,它包含了SENet注意力模块的实现。SENet注意力模块主要由Squeeze操作和Excitation操作组成。
- Squeeze操作:将输入特征图进行全局池化,将每个通道的特征图转化为一个单一的数值。
- Excitation操作:使用全连接层对每个通道的特征进行映射,得到权重向量,表示每个通道的重要性。
- 最后,通过将输入特征图与权重向量进行乘法操作,得到加权后的特征图。
3. 在原始的OpenPose网络中,找到合适的位置插入SEBlock模块。一般来说,可以在每个卷积层(Conv2d)之后添加一个SEBlock模块。
4. 根据具体的网络结构,对每个卷积层后添加SEBlock模块的位置进行修改。可以通过继承nn.Module并重新定义forward函数来实现。
5. 在forward函数中,对每个卷积层后添加SEBlock模块,并将其结果作为输入传递给下一层。
6. 在训练过程中,根据需要进行参数更新和反向传播。
示例代码如下:
```python
import torch
import torch.nn as nn
import torchvision.models as models
# 定义SEBlock模块
class SEBlock(nn.Module):
def __init__(self, in_channels, reduction_ratio=16):
super(SEBlock, self).__init__()
self.squeeze = nn.AdaptiveAvgPool2d(1)
self.excitation = nn.Sequential(
nn.Linear(in_channels, in_channels // reduction_ratio),
nn.ReLU(inplace=True),
nn.Linear(in_channels // reduction_ratio, in_channels),
nn.Sigmoid()
)
def forward(self, x):
batch_size, channels, _, _ = x.size()
squeeze = self.squeeze(x).view(batch_size, channels)
excitation = self.excitation(squeeze).view(batch_size, channels, 1, 1)
weighted_x = x * excitation.expand_as(x)
return weighted_x
# 在OpenPose网络中添加SEBlock模块的实现
class OpenPoseWithSENet(nn.Module):
def __init__(self):
super(OpenPoseWithSENet, self).__init__()
self.backbone = models.resnet18(pretrained=True)
self.se_block1 = SEBlock(64)
self.se_block2 = SEBlock(128)
self.se_block3 = SEBlock(256)
self.se_block4 = SEBlock(512)
def forward(self, x):
x = self.backbone.conv1(x)
x = self.backbone.bn1(x)
x = self.backbone.relu(x)
x = self.backbone.maxpool(x)
x = self.backbone.layer1(x)
x = self.se_block1(x)
x = self.backbone.layer2(x)
x = self.se_block2(x)
x = self.backbone.layer3(x)
x = self.se_block3(x)
x = self.backbone.layer4(x)
x = self.se_block4(x)
return x
# 初始化网络并进行训练
model = OpenPoseWithSENet()
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.001, momentum=0.9)
# 进行训练和测试
for epoch in range(num_epochs):
# 训练代码
# 测试代码
```
以上是在lightweight OpenPose人体姿态估计网络中添加SENet注意力模块的简要描述和代码实现。具体实现过程可能因网络结构和需求的不同而有所变化,需要根据具体情况进行调整和修改。
### 回答3:
在轻量级OpenPose人体姿态估计网络中,我们可以通过添加SENet注意力模块来提升网络的性能。SENet注意力模块通过自适应地学习每个通道的权重,使得网络能够更加关注重要的特征信息。
首先,在网络的每个卷积层之后,我们可以添加一个SENet注意力模块。该模块由一个全局平均池化层和两个全连接层组成。全局平均池化层将特征图压缩成一个特征向量,然后通过两个全连接层获得每个通道的权重。最后,通过乘法操作将权重应用到原始特征图中。
下面是伪代码实现:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class SENet(nn.Module):
def __init__(self, in_channels, reduction_ratio=16):
super(SENet, self).__init__()
self.avg_pool = nn.AdaptiveAvgPool2d(1)
self.fc1 = nn.Linear(in_channels, in_channels // reduction_ratio)
self.fc2 = nn.Linear(in_channels // reduction_ratio, in_channels)
def forward(self, x):
b, c, _, _ = x.size()
y = self.avg_pool(x).view(b, c)
y = F.relu(self.fc1(y))
y = torch.sigmoid(self.fc2(y)).view(b, c, 1, 1)
return x * y
class LightweightOpenPose(nn.Module):
def __init__(self):
super(LightweightOpenPose, self).__init__()
# define your network architecture here
self.conv1 = nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1)
self.senet1 = SENet(64)
self.conv2 = nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1)
self.senet2 = SENet(128)
# add more layers and SENet modules here
def forward(self, x):
x = F.relu(self.senet1(self.conv1(x)))
x = F.relu(self.senet2(self.conv2(x)))
# add more forward operations here
return x
```
在这个示例中,我们只添加了两个SENet模块。你可以根据自己的需求在更多的卷积层之后添加SENet模块。同时,你还可以根据具体任务的需要来调整注意力模块中的超参数,如reduction_ratio等。这个代码只是一个示例,并不是针对具体应用定制的最优实现。
阅读全文
相关推荐
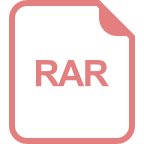
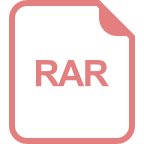
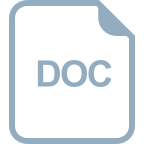
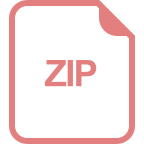
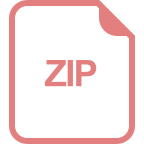
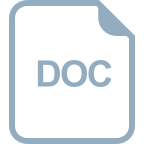
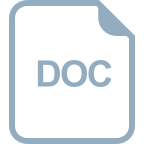
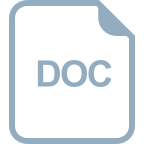
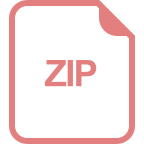
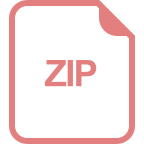
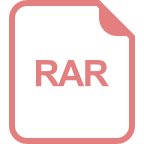
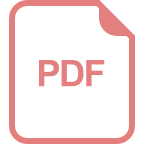
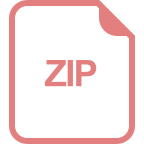
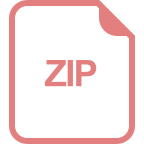
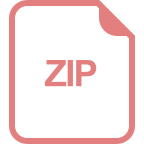
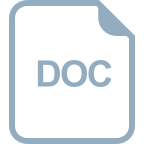
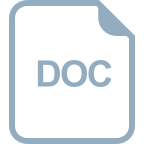