接住任意一种排序算法对某班的Java成绩进行升序排序(冒泡或选择),写出完整代码,要求难度低
时间: 2024-09-26 12:12:32 浏览: 34
当然,我们可以使用简单的冒泡排序算法来对班级的成绩列表进行升序排序。这里是一个使用Java编写的冒泡排序示例:
```java
import java.util.ArrayList;
import java.util.List;
public class StudentScoreSorter {
// 定义一个学生分数类
public static class Score {
private String studentName;
private double score;
public Score(String studentName, double score) {
this.studentName = studentName;
this.score = score;
}
public String getStudentName() {
return studentName;
}
public double getScore() {
return score;
}
}
// 冒泡排序方法
public static void bubbleSort(List<Score> scores) {
int n = scores.size();
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (scores.get(j).getScore() > scores.get(j + 1).getScore()) { // 比较并交换
Score temp = scores.get(j);
scores.set(j, scores.get(j + 1)); // 交换位置
scores.set(j + 1, temp);
}
}
}
}
public static void main(String[] args) {
// 创建一个学生分数列表
List<Score> studentsScores = new ArrayList<>();
studentsScores.add(new Score("Alice", 85.0));
studentsScores.add(new Score("Bob", 92.0));
studentsScores.add(new Score("Charlie", 78.0));
studentsScores.add(new Score("David", 96.0));
System.out.println("Before sorting:");
for (Score score : studentsScores) {
System.out.println(score.getStudentName() + ": " + score.getScore());
}
// 对列表进行排序
bubbleSort(studentsScores);
System.out.println("\nAfter sorting in ascending order:");
for (Score score : studentsScores) {
System.out.println(score.getStudentName() + ": " + score.getScore());
}
}
}
```
这个例子定义了一个`Score`类来表示学生的姓名和成绩,然后在`bubbleSort`方法中实现了冒泡排序算法。在`main`方法中创建了一个学生的成绩列表,并对其进行排序后输出。
阅读全文
相关推荐
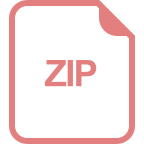
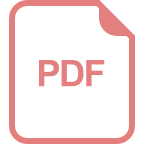
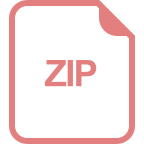















