AES加密解密代码C#
时间: 2024-01-02 07:03:21 浏览: 132
以下是C#实现AES加密解密的代码示例:
1. AES加密代码示例:
```csharp
using System;
using System.Security.Cryptography;
using System.Text;
public class AesEncryption
{
public static string Encrypt(string plainText, string key, string iv)
{
byte[] keyBytes = Encoding.UTF8.GetBytes(key);
byte[] ivBytes = Encoding.UTF8.GetBytes(iv);
using (Aes aes = Aes.Create())
{
aes.Key = keyBytes;
aes.IV = ivBytes;
ICryptoTransform encryptor = aes.CreateEncryptor(aes.Key, aes.IV);
using (var ms = new System.IO.MemoryStream())
{
using (var cs = new CryptoStream(ms, encryptor, CryptoStreamMode.Write))
{
using (var sw = new System.IO.StreamWriter(cs))
{
sw.Write(plainText);
}
}
byte[] encryptedBytes = ms.ToArray();
return Convert.ToBase64String(encryptedBytes);
}
}
}
}
```
2. AES解密代码示例:
```csharp
using System;
using System.Security.Cryptography;
using System.Text;
public class AesDecryption
{
public static string Decrypt(string cipherText, string key, string iv)
{
byte[] keyBytes = Encoding.UTF8.GetBytes(key);
byte[] ivBytes = Encoding.UTF8.GetBytes(iv);
using (Aes aes = Aes.Create())
{
aes.Key = keyBytes;
aes.IV = ivBytes;
ICryptoTransform decryptor = aes.CreateDecryptor(aes.Key, aes.IV);
using (var ms = new System.IO.MemoryStream(Convert.FromBase64String(cipherText)))
{
using (var cs = new CryptoStream(ms, decryptor, CryptoStreamMode.Read))
{
using (var sr = new System.IO.StreamReader(cs))
{
return sr.ReadToEnd();
}
}
}
}
}
}
```
使用示例:
```csharp
string plainText = "Hello, World!";
string key = "1234567812345678"; // Key要16位的
string iv = "@12345678912345!";
string encryptedText = AesEncryption.Encrypt(plainText, key, iv);
string decryptedText = AesDecryption.Decrypt(encryptedText, key, iv);
Console.WriteLine("加密后的密文: " + encryptedText);
Console.WriteLine("解密后的明文: " + decryptedText);
```
阅读全文
相关推荐



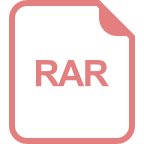








