python txt文本处理
时间: 2023-11-08 18:02:12 浏览: 52
对于Python中的文本处理,可以使用内置的文件操作功能和字符串方法来处理txt文本文件。以下是一些常见的文本处理任务及其对应的Python代码示例:
1. 读取文本文件:
```python
with open('file.txt', 'r') as file:
content = file.read()
```
2. 写入文本文件:
```python
content = "Hello, World!"
with open('file.txt', 'w') as file:
file.write(content)
```
3. 逐行读取文本文件:
```python
with open('file.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line)
```
4. 搜索特定字符串:
```python
content = "Hello, World!"
if "World" in content:
print("Found")
else:
print("Not Found")
```
5. 替换字符串:
```python
content = "Hello, World!"
new_content = content.replace("Hello", "Hi")
print(new_content)
```
6. 分割字符串:
```python
content = "Hello, World!"
words = content.split()
print(words)
```
7. 统计单词数量:
```python
content = "Hello, World!"
words = content.split()
word_count = len(words)
print(word_count)
```
8. 统计特定词出现次数:
```python
content = "Hello, World! Hello, Universe!"
word_to_count = "Hello"
count = content.count(word_to_count)
print(count)
```
相关推荐
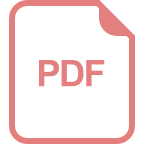
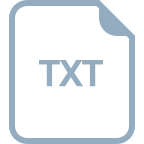














