c语言链栈的入栈出栈读栈顶遍历写在一个程序里
时间: 2024-12-19 12:15:22 浏览: 9
在 C 语言中,我们可以使用链表数据结构来实现一个简单的链式栈。链栈是一种动态数组,它的优点是可以动态地增加或减少存储空间,不需要预先知道栈的最大容量。以下是链栈的基本操作:
1. **入栈(Push)**:
- 定义一个节点结构体(Node),包含数据域 `data` 和指向下一个节点的指针 `next`。
- 创建新的节点,并将其 `data` 设置为新元素,`next` 指向当前栈顶(如果栈为空,则 `next` 指针初始化为 `NULL`)。
- 将新的节点赋值给栈顶指针(`top = new Node; top->data = value; top->next = current_top;`),其中 `current_top` 是当前栈顶。
2. **出栈(Pop)**:
- 如果栈空,返回错误或抛异常。
- 删除栈顶节点,将 `current_top` 更新为 `current_top->next`。
- 返回删除节点的数据 (`return top->data;`)。
3. **读栈顶(Top)**:
- 如果栈空,返回错误或特殊值表示栈顶为空。
- 直接返回栈顶节点的数据 (`return top->data;`)。
4. **遍历(Traversal)**:
- 使用迭代或递归的方式从栈顶开始访问每个元素,直到遍历到栈底。
- 可以创建一个辅助函数,每次调用该函数都取出栈顶元素并处理,然后继续调用自身,直到栈为空。
下面是一个简化的链栈实现示例(使用头插法):
```c
#include <stdio.h>
typedef struct Node {
int data;
struct Node* next;
} StackElement;
StackElement* top = NULL; // 初始化栈顶
// 入栈
void push(int value) {
StackElement* newNode = (StackElement*)malloc(sizeof(StackElement));
if (newNode == NULL) {
printf("Memory allocation failed!\n");
return;
}
newNode->data = value;
newNode->next = top;
top = newNode;
}
// 出栈
int pop() {
if (top == NULL) {
printf("Stack is empty.\n");
return -1; // 或者抛异常
}
StackElement* temp = top;
int data = temp->data;
top = top->next;
free(temp);
return data;
}
// 读栈顶
int peek() {
if (top == NULL) {
printf("Stack is empty.\n");
return -1; // 或者抛异常
}
return top->data;
}
// 遍历
void traverse() {
StackElement* temp = top;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
// 测试入栈、出栈、读栈顶和遍历
push(10);
push(20);
push(30);
printf("Top element: %d\n", peek());
printf("Popped element: %d\n", pop());
traverse();
return 0;
}
```
阅读全文
相关推荐
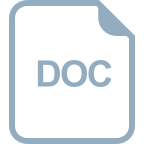
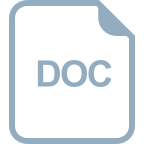
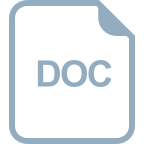


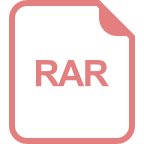
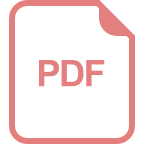
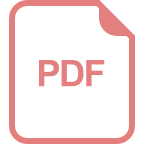








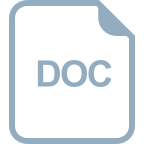
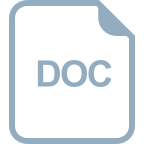
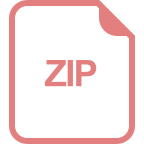